【python】直接使用linux ssh命令在多跳板机情况下设置免密直接登录
本地 --> 跳板机1 --> 跳板机2 -> 目标机器1
ssh-keygen -t rsa 生成公钥和私钥
将公钥写入到所有跳转机的帐户的authorized_keys中实现免密
config文件
HOST 跳板机1jumper
Hostname 100.67.76.9
Port 57822
User root
IdentityFile ~/.ssh/id_rsa
Host 跳板机2
Hostname 10.141.250.1
Port 22
User root
IdentityFile ~/.ssh/id_rsa
ProxyCommand ssh.exe 跳板机1jumper -W %h:%p
Host 目标机器1
Hostname 10.141.35.34
Port 22
User root
IdentityFile ~/.ssh/id_rsa
ProxyCommand ssh.exe 跳板机2 -W %h:%p
Host 目标机器2
Hostname 10.141.1.50
Port 22
User root
IdentityFile ~/.ssh/id_rsa
ProxyCommand ssh.exe 跳板机2 -W %h:%p
mobaxterm工具实现代理免密跳转 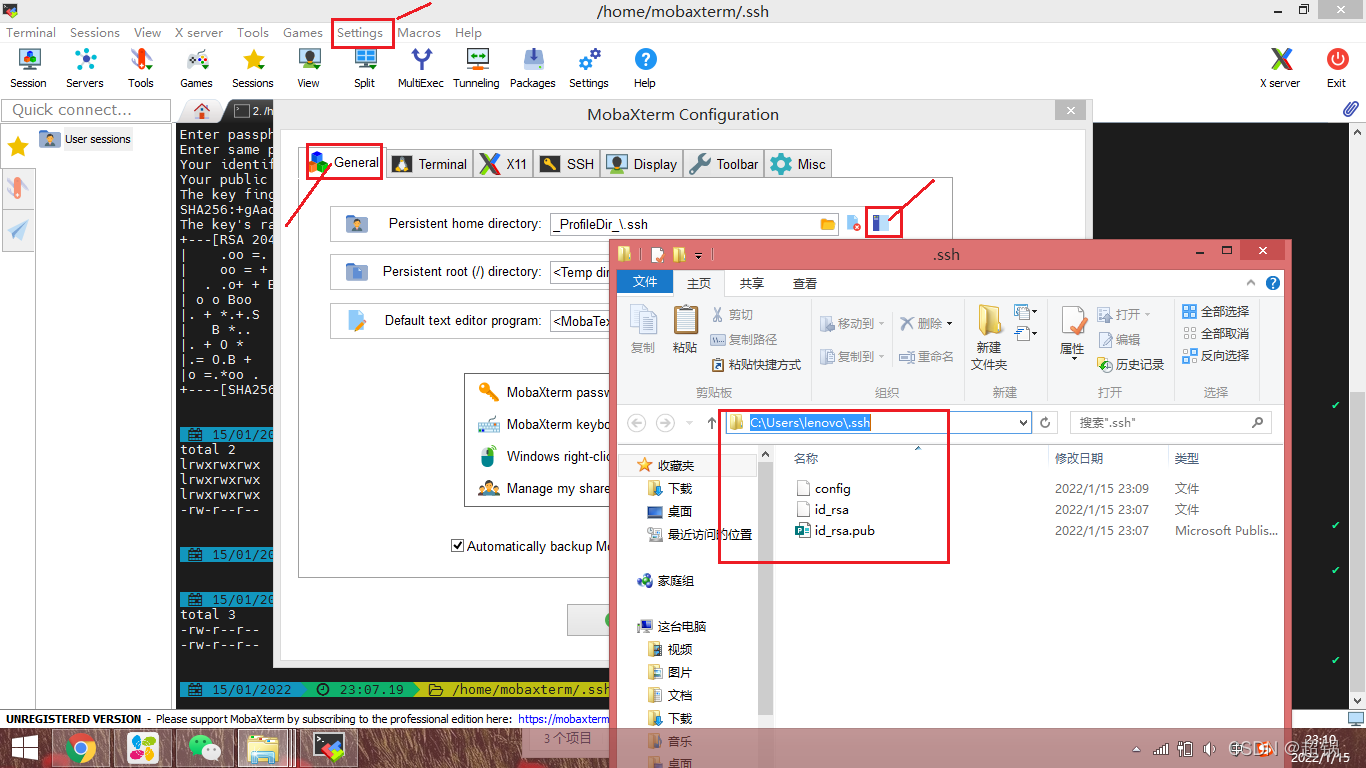
本地 --> 跳板机1 --> 跳板机2 -> 目标机器1
import paramiko
import os
import io
def get_ssh_config():
ssh_config = paramiko.SSHConfig()
user_config_file = os.path.expanduser("~/.ssh/config")
with io.open(user_config_file, 'rt', encoding='utf-8') as f:
ssh_config.parse(f)
return ssh_config
def get_connect_host(host_ssh_config, sock_channel):
key_path = host_ssh_config['identityFile'][0]
private_key = paramiko.RSAKey.from_private_key_file(key_path)
host = paramiko.SSHClient()
host.set_missing_host_key_policy(paramiko.AutoAddPolicy())
host.connect(host_ssh_config['hostname'], port = int(host_ssh_config['port']), username = host_ssh_config['user'], pkey = private_key, sock = sock_channel)
return host
def get_sock_channel(connect_host, dest_ssh_config, local_ssh_config):
transport = connect_host.get_transport()
dest_addr = (dest_ssh_config['hostname'], int(dest_ssh_config['port']))
local_addr = (local_ssh_config['hostname'], int(local_ssh_config['port']))
channel = transport.open_channel("direct-tcpip", dest_addr, local_addr)
return channel
ssh_config = get_ssh_config()
jumper_conf = ssh_config.lookup('跳板机1jumper')
ops_conf = ssh_config.lookup('跳板机2')
gateway_client_conf = ssh_config.lookup('目标机器1')
jumper_host = get_connect_host(jumper_conf, None)
jumper_channel = get_sock_channel(jumper_host, ops_conf, jumper_conf)
ops_host = get_connect_host(ops_conf, jumper_channel)
ops_channel = get_sock_channel(ops_host, gateway_client_conf, ops_conf)
gateway_client = get_connect_host(gateway_client_conf, ops_channel)
stdin, stdout, stderr = gateway_client.exec_command("df -h")
print stdout.read()
gateway_client.close()
ops_host.close()
jumper_host.close()
|