用一台有公网IP地址的云服务器,在其上编写以下脚本:
#!/usr/bin/bash # install frp server in a cloud server
[ $UID -ne 0 ] && (echo "You are not the root, can not excute this script!" && exit 1)
Usage(){ ? ? echo "Usage: $0 -f <frp_package_lcation> -p <install_location> -P <listen_port> -t <token> -d <dashboard_port> ?-u <dashboard_username> -s <dashboard_pwd>" }
PACKAGE="" LOCATION="" PORT="" DUSER="" PASSWD="" TOKEN="" DPORT="" DPASSWD="" while getopts f:p:P:t:d:u:s: arg do ? ? case $arg in ? ? f) ? ? ? ? PACKAGE=$OPTARG ? ? ? ? ;; ? ? p) ? ? ? ? LOCATION=$OPTARG ? ? ? ? ;; ? ? P) ? ? ? ? PORT=$OPTARG ? ? ? ? ;; ? ? t) ? ? ? ? TOKEN=$OPTARG ? ? ? ? ;; ? ? d) ? ? ? ? DPORT=$OPTARG ? ? ? ? ;; ? ? u) ? ? ? ? DUSER=$OPTARG ? ? ? ? ;; ? ? s) ? ? ? ? DPASSWD=$OPTARG ? ? ? ? ;; ? ? ?) ? ? ? ? echo "Invalid Options:~$OPTARG" ? ? ? ? Usage ? ? ? ? exit 1 ? ? ? ? ;; ? ? esac done
# check the package ?whether exists and starts with frp if [ -f ${PACKAGE} ];then ? ? package=$(basename "${PACKAGE}") ? ? if [[ ! "${package}"=~^frp ]] || [[ ?! "{package}"=~\.tar\.gz$ ]];then ? ? ? ? echo "The package is not the frp package, please use the right package!" ? ? ? ? exit 1 ? ? fi else ? ? echo "The package to install was wrong, please check again! " ? ? Usage ? ? exit 1 fi
# check the path to install the package is right if [ -n "${LOCATION}" ];then ? ? LOCATION=${LOCATION%/} ? ? if [ ! -e ${LOCATION} ];then ? ? ? ? mkdir -p ${LOCATION} ? ? ? ? [ $? -ne 0 ] && ( echo "can not make the path to install the package" ?&& exit 2) ? ? elif [ ! -d ${LOCATION} -o ! -x ${LOCATION} ];then ? ? ? ? echo "you did not give the right location or it can not be accessed!" ? ? ? ? exit 2 ? ? fi else ? ? echo "You must give the location to install with -p option argument" ? ? Usage ? ? exit 2 fi
if [ -n "${PORT}" ];then ? ? if [[ ! "${PORT}" =~ ^[0-9]+$ ?]];then ? ? ? ? echo "The Listen port must be a number smaller than 65536" ? ? ? ? exit 3 ? ? fi ? ? if [ ${PORT} -gt 65536 ];then ? ? ? ? echo "The Listen port must be a number smaller than 65536" ? ? ? ? exit 3 ? ? fi ? ? port=$( ss -ltnp | sed -n '2,$p' | awk '{print $4}' | cut -d':' -f2 | grep ${PORT}) ? ? if [ -n "${port}" ];then ? ? ? ? echo "${PORT} is already be used by another program, Please use anther port" ? ? ? ? exit 3 ? ? fi
else ? ? PORT=7000 fi
if [ -n "$TOKEN" ];then ? ? if [[ ! "$TOKEN" =~ ^[a-zA-Z0-9_]{6,10}$ ]];then ? ? ? ? echo "token can only contain alphabeta , digital or underscore from 6 to 10" ? ? ? ? exit 4 ? ? fi else ? ? echo "You must give the token consisting of only alphabeta, digital or underscore from 6 to 10 using -t option" ? ? Usage ? ? exit 4 fi
if [ -n "$DPORT" ];then ? ? if [[ ! "${DPORT}" =~ ^[0-9]+$ ?]];then ? ? ? ? echo "The Listen port must be a number smaller than 65536" ? ? ? ? exit 3 ? ? fi ? ? if [ ${DPORT} -gt 65536 ];then ? ? ? ? echo "The Listen port must be a number smaller than 65536" ? ? ? ? exit 3 ? ? fi ? ? port=$( ss -ltnp | sed -n '2,$p' | awk '{print $4}' | cut -d':' -f2 | grep ${DPORT}) ? ? if [ -n "${port}" -o "${port}" == "${PORT}" ];then ? ? ? ? echo "${DPORT} is already be used by another program, Please use anther port" ? ? ? ? exit 3 ? ? fi
else ? ? DPORT=7500 fi
if [ -n "DUSER" ];then ? ? if [[ ! "${DUSER}" =~ ^[a-zA-Z]{1}[a-zA-Z0-9_]{2,7}$ ]];then ? ? ? ? echo "dash board username should start with alphabeta and only contain alphabeta, digital or underscore from 3 to 8 characters" ? ? ? ? exit 5 ? ? fi else ? ? ? ? echo "You shold give dash board username ?starting with alphabeta and only containing alphabeta, digital or underscore from 3 to 8 characters" ? ? ? ? Usage ? ? ? ? exit 5 fi
if [ -n "$DPASSWD" ];then ? ? if [[ ! "$DPASSWD" =~ ^[a-zA-Z0-9_]{6,10}$ ]];then ? ? ? ? echo "dash board password can only contain alphabeta , digital or underscore from 6 to 10" ? ? ? ? exit 6 ? ? fi else ? ? echo "You must give the dash board password consisting of only alphabeta, digital or underscore from 6 to 10 using -s option" ? ? Usage ? ? exit 6 fi
echo "Package Location:$PACKAGE" echo "Install Location:$LOCATION" echo "Listen Port:$PORT" echo "Token:$TOKEN" echo "Dash board Port:$DPORT" echo "Dash board User:$DUSER" echo "Dash board Password:$DPASSWD"
read -p "Are you Sure to use these above arguments[Yes/No]" CHOSE
if [[ ?! "$CHOSE" =~ ^[Yy]es$ ]];then ? ? echo "You did not choose to install the package, exit" ? ? exit 1 fi
echo "Starting to install frp package ..." echo "uncompress the ${PACKAGE} to ${LOCATION} ..." tar -xvzf ${PACKAGE} -C ${LOCATION} &>/dev/null [ $? -ne 0 ] && (echo "can not uncompress the package to destination" && exit 1) echo "uncompressed ?completed"
CURDIR=$(pwd) package=$(basename ${PACKAGE}) INSTDIR=${LOCATION}/${package%.tar.gz}
echo "Goto to install path: ${INSTDIR}" cd ${INSTDIR} || (echo "can not access the path: ${INSTDIR}" && exit 1) echo "backup frps.ini to frp.ini.bak" mv frps.ini frps.ini.bak [ $? -ne 0 ] && (echo "backup failed" && exit 1)
echo "Edit the server configuration: " cat >frps.ini<<EOF [common] bind_port = ${PORT} # this token will be used by clients token =${TOKEN}
dashboard_port = ${DPORT} # frp background manager will use this username and password dashboard_user = ${DUSER} dashboard_pwd = ${DPASSWD} enable_prometheus = true
# frp log configuration log_file = /var/log/frps.log log_level = info log_max_days = 3 EOF echo "Edit finished!"
CONFIGDIR=/etc/frp
if [ ! -d /etc/frp ];then ? ? mkdir -p "${CONFIGDIR}" ? ? [ $? -ne 0 ] && (echo "can not make the configuration path:${CONFIGDIR}" && exit) fi cp frps.ini /etc/frp cp frps /usr/bin cp systemd/frps.service /usr/lib/systemd/system/ systemctl enable frps systemctl start frps
firewall-cmd --permanent --add-port=${PORT}/tcp firewall-cmd --permanent --add-port=${DPORT}/tcp firewall-cmd --reload
echo "back to the path: ${CURDIR}" cd ${CURDIR} echo "Install and start the frp package successfully!"
按如下执行以上编写好的脚本,成功安装frp软件包:
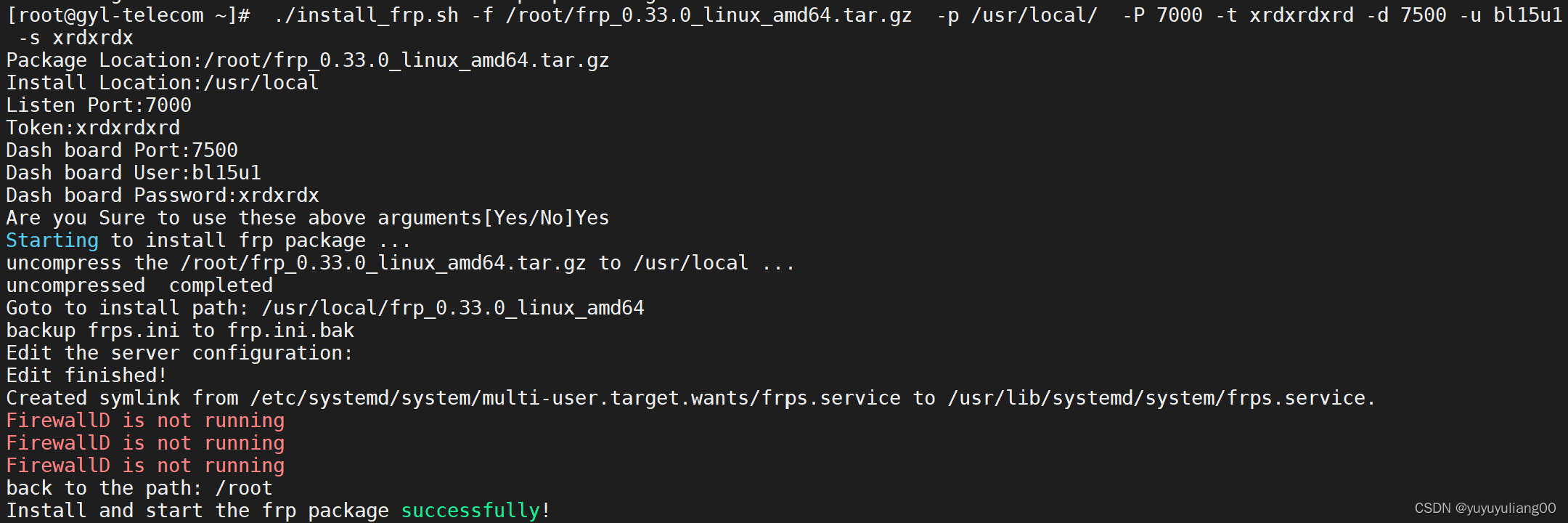
在云主机上输入:ss -tnlp 可以看到服务器已经监听指定的tcp端口7000和7500
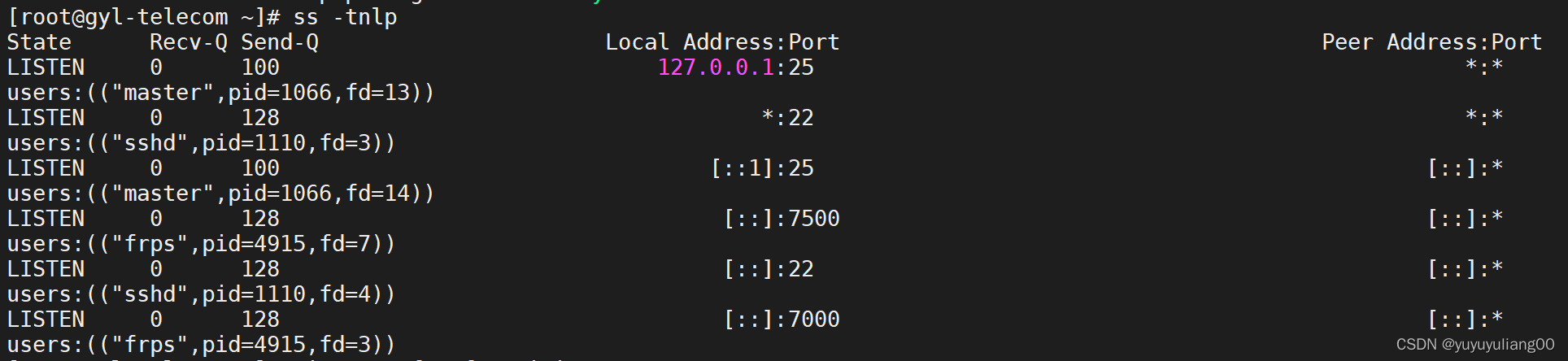
?
在一台能够连接互联网的计算机上,打开一个浏览器,输入:<IP地址>:7500,frp的后台管理客户端成功启动:
注意:在云主机的安全组规则中需要放行访问7000和7500的TCP端口的入方向。
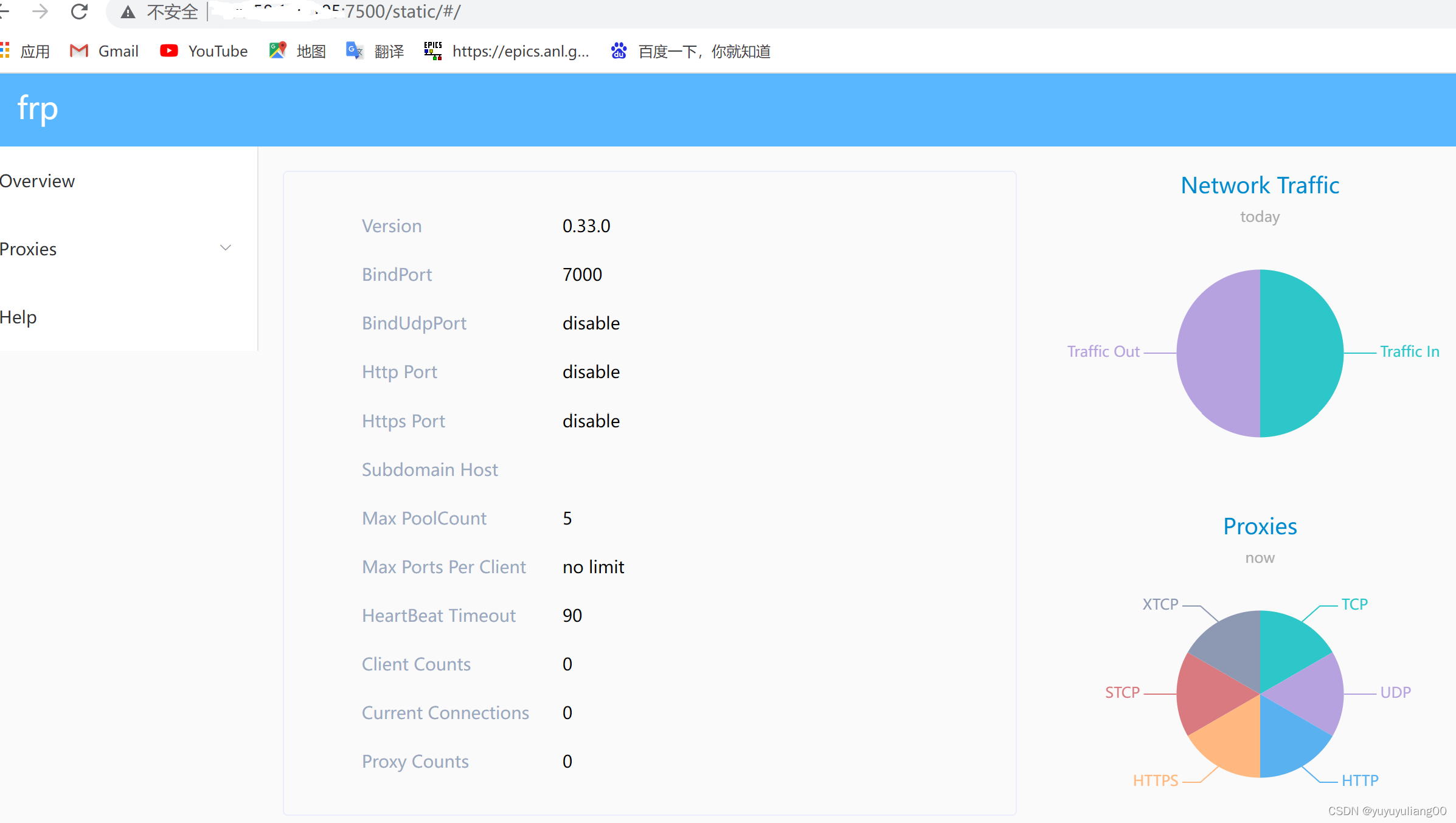
?
?
?
|