pyautogui
官方文档速览 https://pyautogui.readthedocs.io/en/latest/index.html 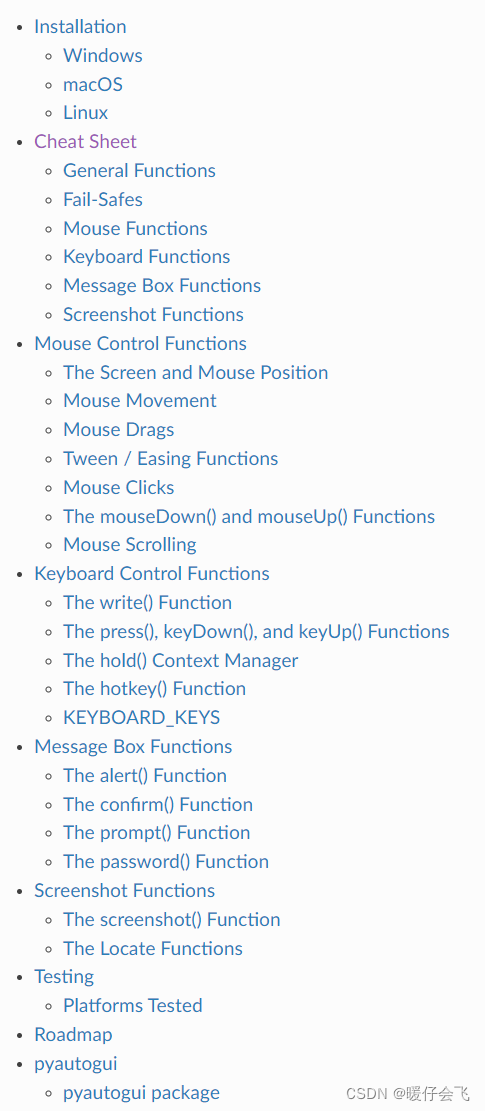
获取鼠标位置
能够实现下面功能:
import pyautogui as pg
pos = pg.position()
print(pos.x,pos.y)
键盘相关
pg.typewrite("hello")
pg.press('1')
- 程序睡眠指定的时间,类似于 time.sleep()
pg.sleep(10)
print("hello")
鼠标相关
pg.moveTo((35,50))
pg.click()
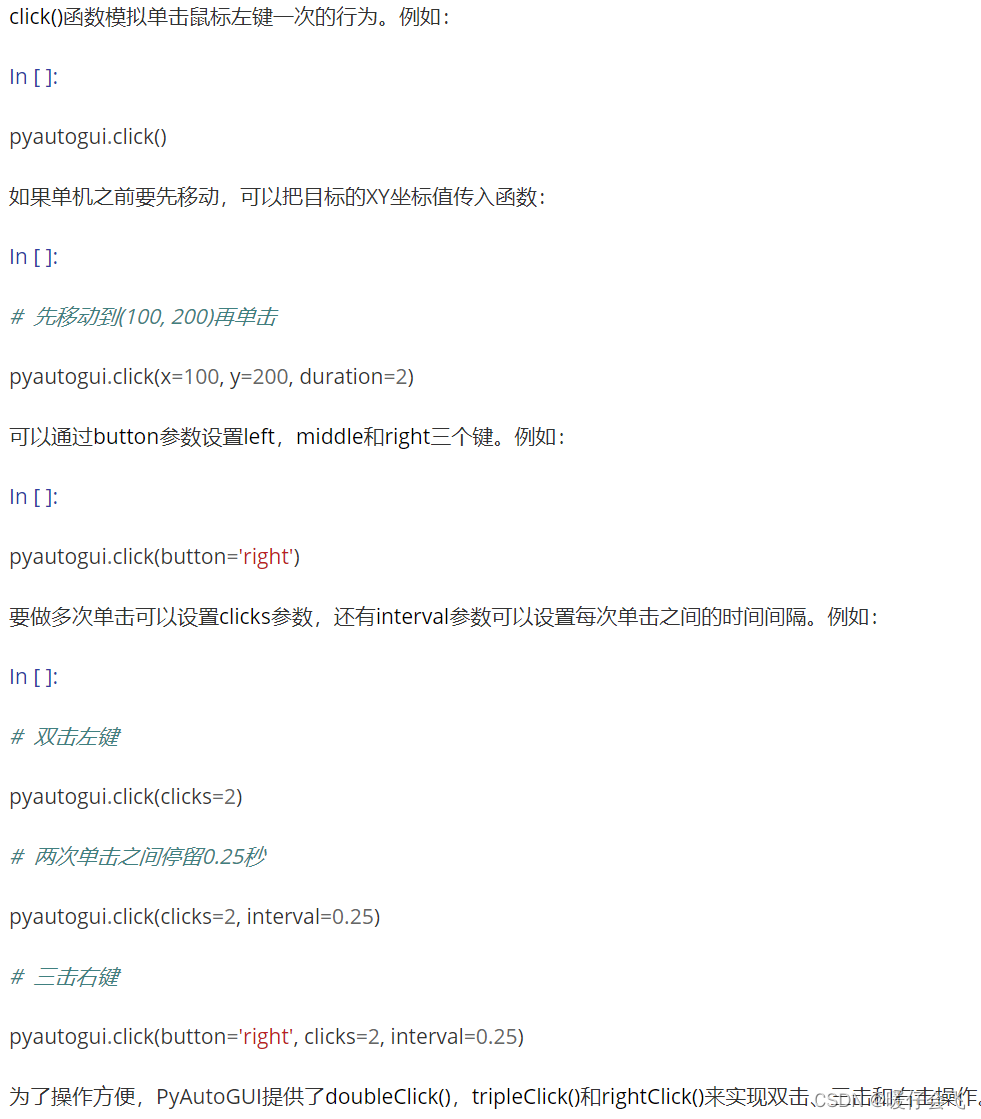
通过图片定位位置
'''遮挡住图标不行,用扩展屏幕也不行'''
pos = pg.locateAllOnScreen("./img.png")
for p in pos:
print(p)
D:\Anaconda3\envs\data\python.exe G:/Gait_Reproduce/t.py
Box(left=1277, top=368, width=90, height=58)
Process finished with exit code 0
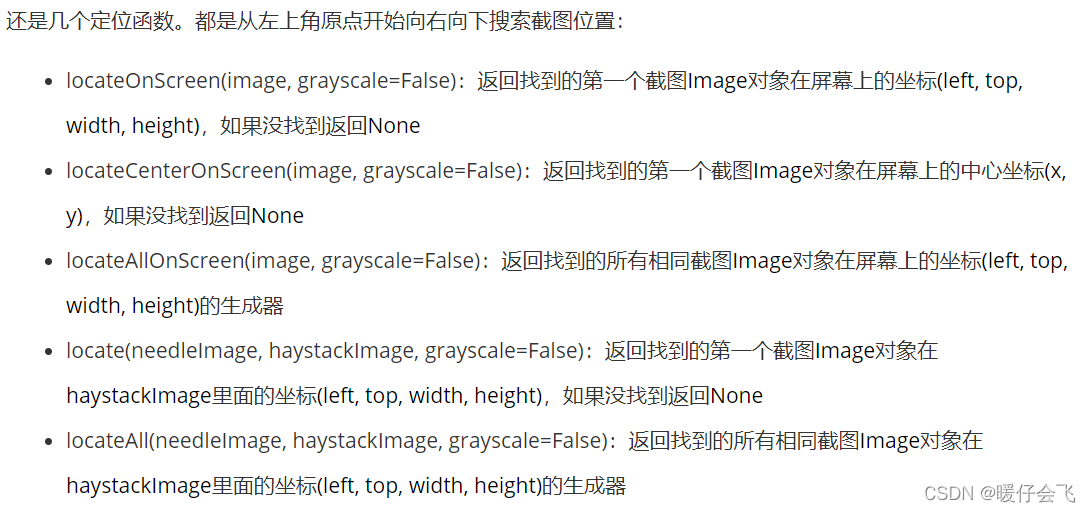
参考页面 https://www.jianshu.com/p/8e508c6a05ce https://www.pianshen.com/article/7555171409/
keyboard
专门的 API 文档如下: https://github.com/boppreh/keyboard#api
能够实现下述功能:
while True:
if k.is_pressed('enter'):
print("1 is pressed")
- 等待某个键按下之后再操作后面的代码
当 “1” 按下之前,不会运行下面的 “hello” 代码
k.wait('1')
print("hello")
while True:
k.press('1')
'''首先确定一个事件,通过这个事件来触发内容'''
event = k.KeyboardEvent("down",123,'space')
'''通过一个函数来指定处理策略,这个传入的 x 的类型就是一个 KeyboardEvent 对象,所以要与我们设定的这个对象进行匹配'''
def reply_press(x):
if x.event_type == event.event_type and x.name == event.name:
print("pressed down the space")
'''通过 hook 操作,将这些针对某个事件的响应操作 “挂到” 键盘上'''
k.hook(reply_press)
'''等待事件触发,但是后面写的代码内容阻塞住不执行,如果不想让程序阻塞的话,可以不使用 wait'''
for i in range(100):
time.sleep(0.5)
print(i)
'''不使用 wait 后面的程序会依次执行,过程中 hook 指定的响应操作也有效,例如'''
0
1
2
pressed down the space
3
4
5
6
7
8
pressed down the space
9
10
11
12
pressed down the space
13
14
15
pressed down the space
16
Process finished with exit code -1
小训练
- 通过 keyboard 和 pyautogui 库实现一个小功能:
- 通过空格来不断获取鼠标所处位置的值
- 不输入空格的时候不获得鼠标位置
写法1:
while True:
if k.is_pressed('space'):
pos = pg.position()
print(pos.x,pos.y)
写法2:
def generate_position(x):
event = k.KeyboardEvent("down",190,'space')
if x.event_type == event.event_type and x.name == event.name:
pos = pg.position()
print(pos.x,pos.y)
k.hook(generate_position)
for i in range(100):
pg.sleep(1)
print(i)
0
1
2682 622
2
3
4
5
6
3516 254
7
8
9
2668 1015
10
11
2668 1015
12
13
3030 559
14
15
Process finished with exit code -1
|