一、目录操作与文件属性
1、读取目录
>opendir
opendir 函数用来打开 一个目录文件 :
#include <dirent.h>
DIR *opendir(const char *name);
DIR *fdopendir(int fd);
成功 时返回目录流指针 ;出错 时返回NULL
fdopendir 使用文件描述符 ,要配合open函数 使用DIR 是用来描述一个打开的目录文件 的结构体类型
>readdir
readdir 函数用来读取 目录流中的内容 :
#include <dirent.h>
struct dirent *readdir(DIR *dirp);
成功 时返回目录流dirp 中下一个 目录项;出错 或到末尾 时时返回NULL
struct dirent 是用来描述目录流 中一个目录项 的结构体类型 包含成员char d_name[256] 见参考帮助文档
>closedir
closedir 函数用来关闭 一个目录文件:
#include <dirent.h>
int closedir(DIR *dirp);
成功 时返回0 ;出错 时返回EOF(-1)
操作示例: 打印指定的目录下所有文件名称
int main(int argc, char *argv[])
{
{
DIR *dirp;
struct dirent *dp;
if (argc < 2)
{
printf(“Usage : %s <directory>\n”, argv[0]);
return -1;
}
if ((dirp = opendir(argv[1])) == NULL)
{
perror(“opendir”);
return -1;
}
while ((dp = readdir(dirp)) != NULL)
{
printf(“%s\n”, dp->d_name);
}
closedir(dirp);
2、修改文件访问权限
chmod/fchmod 函数用来修改文件 的访问权限 :
#include <sys/stat.h>
int chmod(const char *path, mode_t mode);
int fchmod(int fd, mode_t mode);
成功 时返回0 ;出错 时返回EOF(-1)
root 和文件所有者 能修改文件的访问权限 示例: chmod(“test.txt”, 0666);
注意: 在vmware和windows共享的文件夹下,有些权限不能改变。
3、获取文件属性
stat/lstat/fstat 函数用来获取文件属性 :
#include <sys/stat.h>
int stat(const char *path, struct stat *buf);
int lstat(const char *path, struct stat *buf);
int fstat(int fd, struct stat *buf);
成功 时返回0 ;出错 时返回EOF(-1)
如果path 是文件位置 ,stat 获取的是目标文件 的属性;而lstat 获取的是链接文件 的属性.
struct stat 是存放文件属性的结构体类型 : 重点关注的:
mode_t st_mode;
uid_t st_uid;
uid_t st_gid;
off_t st_size;
time_t st_mtime;
所有成员:
struct stat
{
dev_t st_dev;
ino_t st_ino;
mode_t st_mode;
nlink_t st_nlink;
uid_t st_uid;
gid_t st_gid;
dev_t st_rdev;
off_t st_size;
unsigned long st_blksize;
unsigned long st_blocks;
time_t st_atime;
time_t st_mtime;
time_t st_ctime;
};
文件访问权限 – st_mode
通过系统提供的宏来判断文件类型:
S_IFMT 0170000 文件类型的位遮罩
S_ISREG(st_mode) 0100000 是否常规文件
S_ISDIR(st_mode) 0040000 是否目录
S_ISCHR(st_mode) 0020000 是否字符设备
S_ISBLK(st_mode) 0060000 是否块设备
S_ISFIFO(st_mode) 0010000 是否FIFO文件
S_ISLNK(st_mode) 0120000 是否链接文件
S_ISSOCK(st_mode) 0140000 是否SOCKET文件
if(S_ISREG(buf.st_mode))
{
}
通过系统提供的宏来获取文件访问权限:
S_IRUSR 00400 bit:8 所有者有读权限
S_IWUSR 00200 7 所有者拥有写权限
S_IXUSR 00100 6 所有者拥有执行权限
S_IRGRP 00040 5 群组拥有读权限
S_IWGRP 00020 4 群组拥有写权限
S_IXGRP 00010 3 群组拥有执行权限
S_IROTH 00004 2 其他用户拥有读权限
S_IWOTH 00002 1 其他用户拥有写权限
S_IXOTH 00001 0 其他用户拥有执行权限
for(i = 8;i >= 0; i--)
{
if(buf.st_mode & (1<<i))
{
switch(i%3)
{
case 2:
printf("r");
break;
case 1:
printf("w"); 7、4、1-w
break;
case 0:
printf("x");6、3、0-x
break;
}
}
else
{
printf("-");
}
}
二、库
1、静态库
1.编写库文件 代码,编译为.o 目标文件。 2.ar 命令 创建 libxxxx.a 文件
ar -rsv libxxxx.a xxxx.o
注意:
静态库 名字要以lib 开头,后缀名为.a - 没有
main函数 的.c 文件不能生成可执行文件 。
3.链接静态库:
gcc -o 目标文件 源码.c -L路径 -lxxxx
ar 的参数:
c :禁止在创建库时产生的正常消息r :如果指定的文件已经存在于库中,则替换 它s :无论 ar 命令是否修改了库内容都强制重新生成库符号表v :将建立新库的详细的逐个文件的描述 写至标准输出q :将指定的文件添加到库的末尾t :将库的目录写至标准输出
查看库中符号信息
$nm libhello.a
hello.o:
0000000 T hello
U puts
nm : 显示指定文件 中的符号信息 -a :显示所有符号
2、动态库
1.生成与位置无关代码的目标文件
gcc -c -fPIC xxx.c xxxx.c …
2.生成动态库
gcc -shared -o libxxxx.so xxx.o xxx.o …
3.编译可执行文件
gcc -o 目标文件 源码.c -L路径 -lxxxx
执行动态库的可执行文件错误
./test: error while loading shared libraries: libmyheby.
so: cannot open shared object file:
No such file or directory
含义:可执行文件所使用的动态库找不到
解决办法:
①找到动态库,添加到/usr/lib里面
②使用export LD_LIBRARY_PATH=文件路径:
③动态库目录
添加在~/.bashrc 文件里面
使用source ~/.bashrc 生效。
查看可执行文件使用的动态库:
ldd 命令 : ldd 你的可执行文件
例如:
root@haas-virtual-machine:/mnt/hgfs/share/newIOP# ldd test
linux-vdso.so.1 => (0x00007fff6548d000)
libmyheby.so => /mnt/hgfs/share/newIOP/day5/libmyheby.so (0x00007f5c89521000)
libc.so.6 => /lib/x86_64-linux-gnu/libc.so.6 (0x00007f5c89144000)
/lib64/ld-linux-x86-64.so.2 (0x000055fe52211000)
root@haas-virtual-machine:/mnt/hgfs/share/newIOP/day5# ldd test
linux-vdso.so.1 => (0x00007ffcb652c000)
libmyheby.so => not found
libc.so.6 => /lib/x86_64-linux-gnu/libc.so.6 (0x00007fbeeffaf000)
/lib64/ld-linux-x86-64.so.2 (0x0000561003c3b000)
到这里就结束啦! 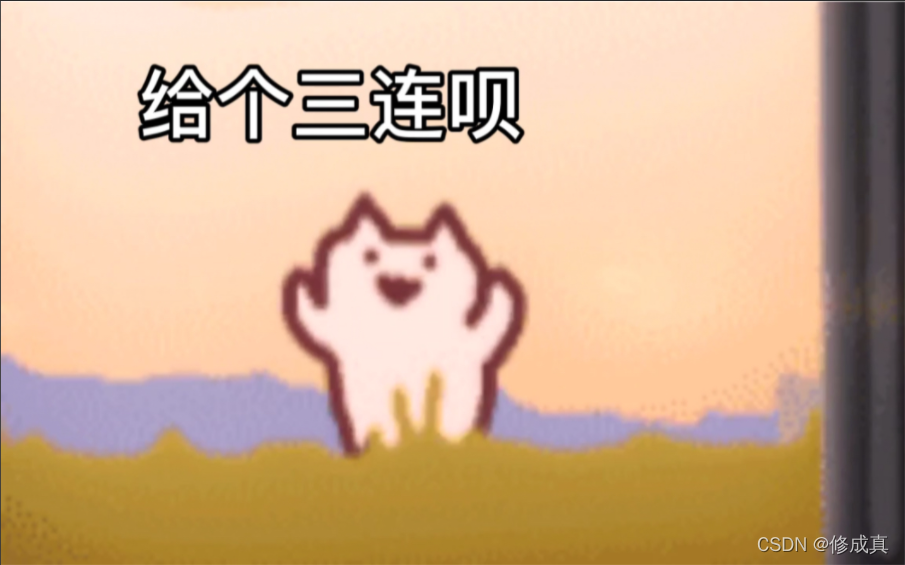
|