概述
本片文章将会主要介绍四个IO函数:开关读写
open函数
- open 函数的输入参数是
open(char* ,flag ,[mode]) - 第一个参数表示的是打开文件的路径文件名
- 第二个参数表示的是打开的方式,见下表
打开方式 | 描述 |
---|
O_RDONLY | 只读 | O_WRONLY | 只写 | O_RDWR | 读写 | O_CREAT | 创建 | O_EXCL | 文件不存在则创建(可以防止重复创建) | O_TRUNC | 擦除文件内容再打开 | O_APPEND | 追加方式打开(不会清除文件内容) | - 第三个参数表示的是文件的权限,但是注意
输入权限要经过umask掩码掩盖 - 返回值:文件创建失败返回
-1 ,创建成功返回文件ID(文件描述符)
通过open函数来实现touch
#include "stdio.h"
#include "unistd.h"
#include "fcntl.h"
int main(int argc ,char *agrv[]){
int fd;
fd = open("./file_been_touched",O_CREAT|O_EXCL,0777);
if(fd<0){
printf("failed");
return -1;
}
else{
printf("sucess");
return 0;
}
}
write函数
read函数和lseek函数
-
读函数有三个参数:从哪里读,读到哪里去,读多少 , read(fd,buf,size) -
当直接去读文件中的内容的时候,由于文件指针的偏移,一般情况下是读不到内容的,需要借助lseek 函数 -
lseek函数有3个参数文件指针,偏移量,当前位置 -
lseek成功的返回值是文件的当前位置
当前位置参数 | 描述 |
---|
SEEK_SET | 当前为文件开头 | SEEK_CUR | 当前为文件指针 | SEEK_END | 当前为文件结尾 |
使用文件操作函数实现cp命令
#include "stdio.h"
#include "unistd.h"
#include "fcntl.h"
#include "string.h"
int main(int argc,char *argv[]){
int rd_fd,wr_fd;
char read_buf[128]={0};
int rd_ret=0;
if(argc<3){
printf("please input src file and des file\n");
return -1;
}
rd_fd=open(argv[1],O_RDONLY);
if(rd_fd<0){
printf("open failure");
return -2;
}
printf("open success\n");
wr_fd=open(argv[2],O_WRONLY);
if(wr_fd<0){
printf("open failure");
return -3;
}
printf("open success");
while(1){
rd_ret=read(rd_fd,read_buf,128);
if(rd_ret<128){
break;
}
write(wr_fd,read_buf,rd_ret);
}
memset(read_buf,0,128);
write(wr_fd,read_buf,rd_ret);
close(rd_fd);
close(wr_fd);
}
diff函数
- 比较文件之间的差异
diff file1 file2
文件IO和标准IO
标准IO中的相关函数不仅可以读写普通文件,也还可以向标准输入输出中读或写 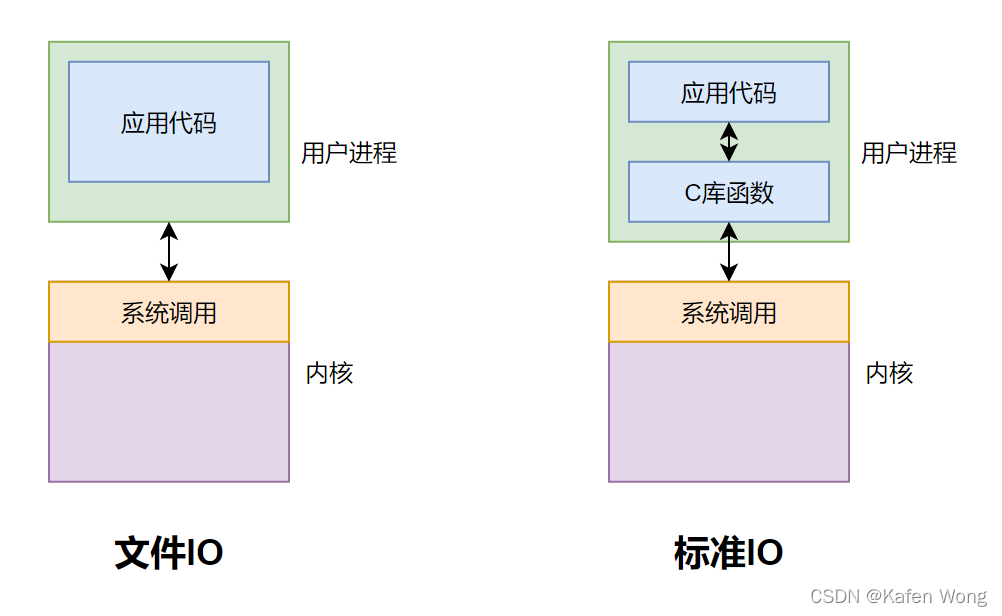
三个buffer
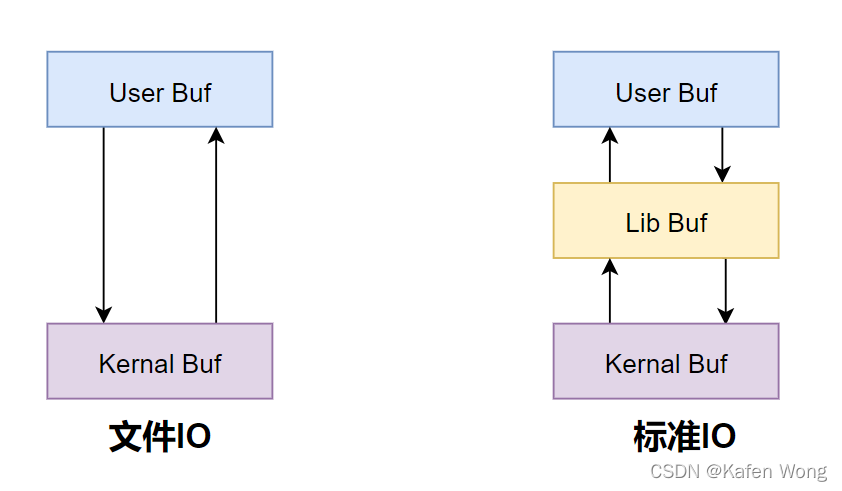
关于printf
- printf遇到
\n 时会将全体缓存的内容谢翱内核中,即调用了系统调用函数 - 库缓存写满时,会把lib buffer的内容写到kernel buf
|