以下部分内容参考博文: https://blog.csdn.net/weixin_50438937/article/details/113630094?spm=1001.2014.3001.5502
一、编程实现人脸识别第一次
 未修改代码:
#include <stdio.h>
#include <curl/curl.h>
typedef unsigned int bool;
#define true 1
#define false 0
bool getUrl(char *filename)
{
CURL *curl;
CURLcode res;
FILE *fp;
if ((fp = fopen(filename, "w")) == NULL)
return false;
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Accept: Agent-007");
curl = curl_easy_init();
if (curl)
{
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
curl_easy_setopt(curl, CURLOPT_URL,"http://www.baidu.com");
curl_easy_setopt(curl, CURLOPT_WRITEDATA, fp);
curl_easy_setopt(curl, CURLOPT_HEADERDATA, fp);
res = curl_easy_perform(curl);
if (res != 0) {
curl_slist_free_all(headers);
curl_easy_cleanup(curl);
}
fclose(fp);
return true;
}
}
bool postUrl(char *filename)
{
CURL *curl;
CURLcode res;
FILE *fp;
if ((fp = fopen(filename, "w")) == NULL)
return false;
curl = curl_easy_init();
if (curl)
{
curl_easy_setopt(curl, CURLOPT_COOKIEFILE, "/tmp/cookie.txt");
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "&logintype=uid&u=xieyan&psw=xxx86");
curl_easy_setopt(curl, CURLOPT_URL, " http://mail.sina.com.cn/cgi-bin/login.cgi ");
curl_easy_setopt(curl, CURLOPT_WRITEDATA, fp);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
fclose(fp);
return true;
}
int main(void)
{
getUrl("/tmp/get.html");
postUrl("/tmp/post.html");
}
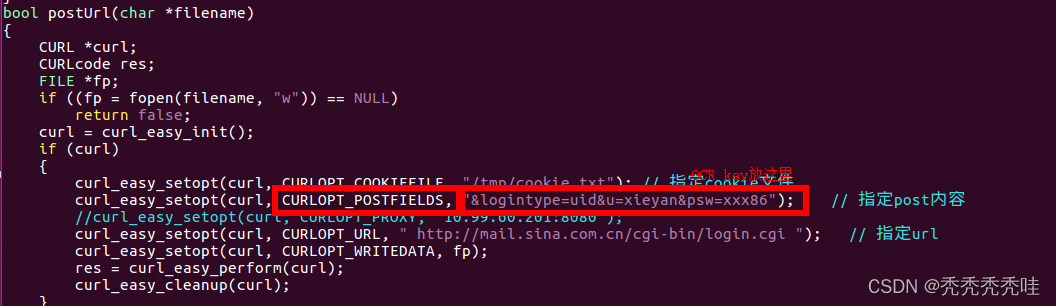 修改后的代码:
#include <stdio.h>
#include <curl/curl.h>
#include <string.h>
#include <stdlib.h>
typedef unsigned int bool;
#define true 1
#define false 0
size_t readData(void *ptr, size_t size, size_t nmemb, void *stream)
{
char buf[1024] = {'\0'};
strncpy(buf,ptr,1024);
printf("===========get data ===========\n");
printf("%s\n",buf);
}
bool postUrl()
{
CURL *curl;
CURLcode res;
char* postString;
char* img1 = NULL;
char* img2 = NULL;
char* key = "xxx";
char* secret = "xxx";
int typeId = 21;
char* format = "xml";
postString = (char* )malloc(strlen(key)+strlen(secret)+2048);
sprintf(postString,"img1=%s&img2=%s&key=%s&secret=%s&typeId=%d&format=%s","","",key,secret,typeId,format);
curl = curl_easy_init();
if(curl){
curl_easy_setopt(curl, CURLOPT_COOKIEFILE, "/tmp/cookie.txt");
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, postString);
curl_easy_setopt(curl, CURLOPT_URL, "https://netocr.com/api/faceliu.do");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION,readData);
res = curl_easy_perform(curl);
printf("OK:%d\n",res);
curl_easy_cleanup(curl);
}
return true;
}
int main(void)
{
postUrl();
}
输入指令:
gcc Test2.c -I ./curl-7.71.1/_install/include/ -L ./curl-7.71.1/_install/lib/ -lcurl

base 64
Base64是网络上最常见的用于传输8Bit字节码的编码方式之一,Base64就是一种基于64个可打印字符来表示二进制数据的方法。可查看RFC2045~RFC2049,上面有MIME的详细规范。 Base64编码是从二进制到字符的过程,可用于在HTTP环境下传递较长的标识信息。采用Base64编码具有不可读性,需要解码后才能阅读。 Linux中查看base64
base64 meinv.png
 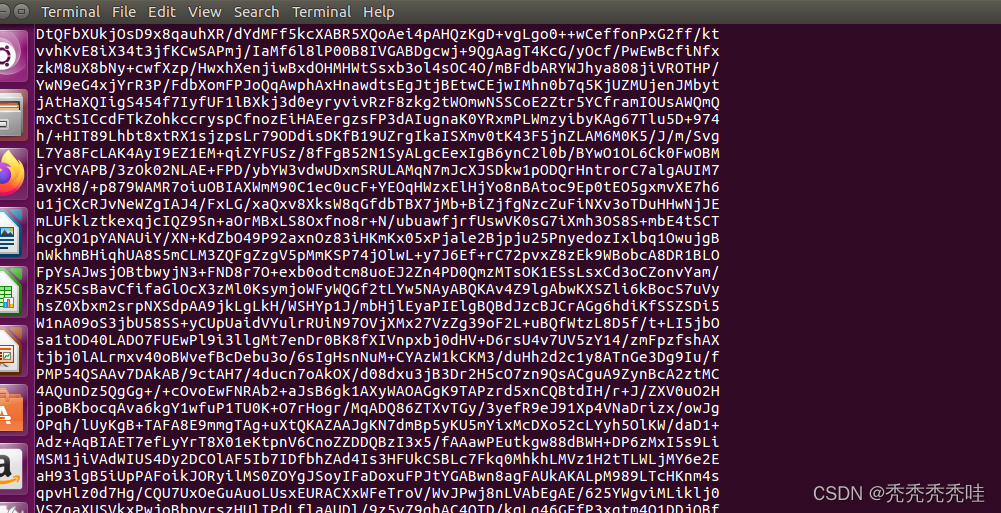
二、加入比对图片
实现代码
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <fcntl.h>
#include <curl/curl.h>
#define true 1
#define false 0
typedef unsigned int bool;
char* faceRes = NULL;
char* getBase64(char* photoPath){
char cmd[256] = {'\0'};
sprintf(cmd, "base64 %s > photoBase64File", photoPath);
system(cmd);
int fd,size;
fd = open("./photoBase64File", O_RDWR);
size = lseek(fd, 0, SEEK_END) + 1;
lseek(fd, 0, SEEK_SET);
char* res = (char*)malloc(size);
memset(res, '\0', size);
read(fd, res, size);
close(fd);
system("rm photoBase64File");
return res;
}
size_t handle(void *ptr, size_t size, size_t nmemb, void *stream){
int ssize = strlen(ptr) + 1;
faceRes = (char*)malloc(ssize);
memset(faceRes, '\0', ssize);
strncpy(faceRes, ptr, ssize);
}
bool postUrl()
{
CURL *curl;
CURLcode res;
char* message = NULL;
char* img1 = getBase64("./photo1.jpg");
char* img2 = getBase64("./photo2.jpg");
char* key = "用自己的";
char* secret = "用自己的";
int typeId = 21;
char* format = "xml";
int size = strlen(img1)+strlen(img2)+strlen(key)+strlen(secret)+strlen(format)+3;
message = (char*)malloc(size);
memset(message, '\0', size);
sprintf(message, "&img1=%s&img2=%s&key=%s&secret=%s&typeId=%d&format=%s",
img1, img2, key, secret, typeId, format);
curl = curl_easy_init();
if (curl)
{
curl_easy_setopt(curl, CURLOPT_URL, "https://netocr.com/api/faceliu.do");
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, message);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, handle);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
if(res != 23){
printf("post failed!\n");
return false;
}else{
printf("post successful!\n");
}
printf("%s\n",faceRes);
if(strstr(faceRes, "是") != NULL){
printf("This is the same person!\n");
}else{
printf("Tow different persons!\n");
}
free(faceRes);
free(img1);
free(img2);
}
int main(void)
{
postUrl();
return 0;
}
gcc demo1.c -I ./curl-7.71.1/_install/include/ -L ./curl-7.71.1/_install/lib/ -lcurl
输出结果: 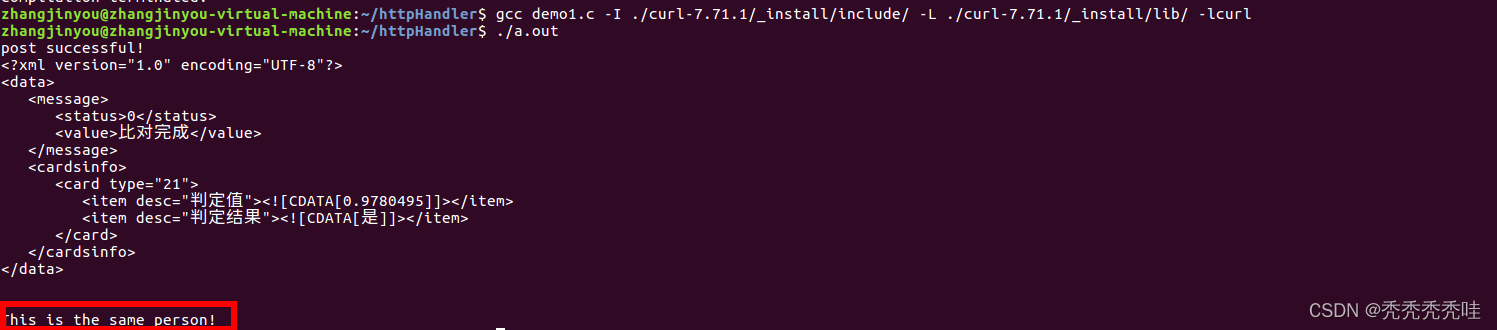 photo1: 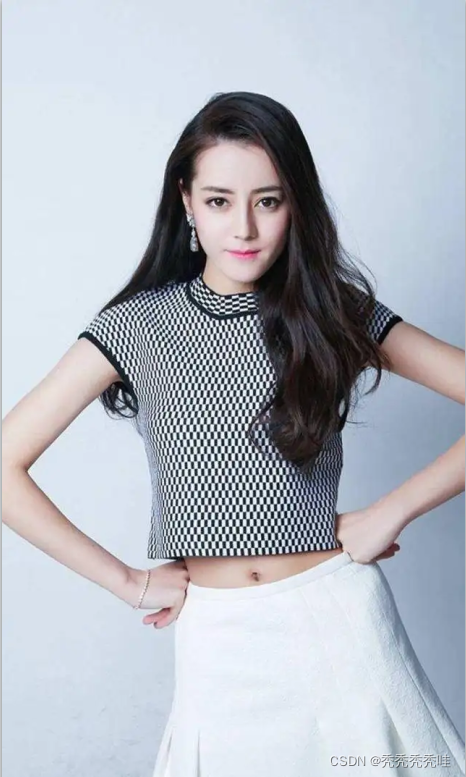 photo2: 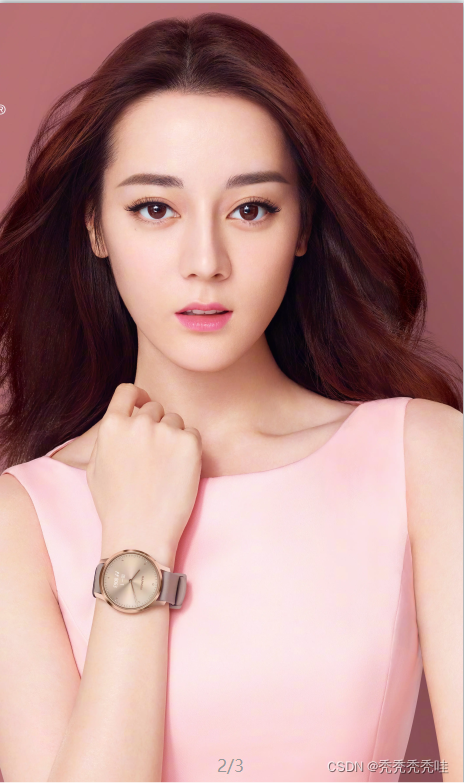
|