实验目的: 1.通过Python实现对底层硬件信息的获取 (包括但不限于 CPU架构、CPU核数、内存信息、磁盘信息、 网络信息) 实验内容: 1.查看-CPU架构 2.查看-CPU核数 3.查看-内存信息 4.查看-磁盘信息 5.查看-网络信息
1)查看-CPU架构
#Python中,platform模块给提供了很多方法去获取操作系统的信息。
#encoding:utf-8
import platform
print(platform.platform()) #获取操作系统名称及版本号
print(platform.version()) #获取操作系统版本号
print(platform.architecture()) #获取操作系统的位数
print(platform.machine()) #计算机的网络名称
print(platform.processor()) #计算机处理器信息
print(platform.uname()) #包含上面所有的信息汇总
示例如下: kali Linux: 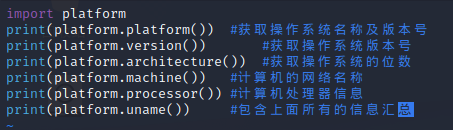 CentOS 7: 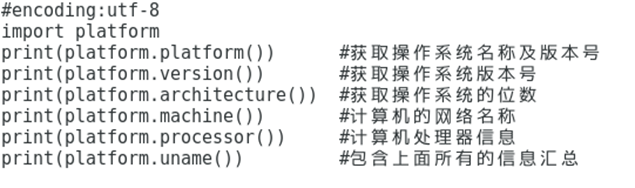 输出结果: 

2)查看-CPU核数
#encoding:utf-8
import multiprocessing
print(multiprocessing.cpu_count())
示例如下: kali Linux:  CentOS 7: 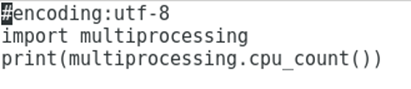 输出结果: 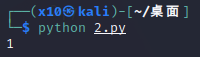

3)查看-内存信息
#encoding:utf-8
mem_info = ""
f_mem_info =open("/proc/meminfo")
try:
for line in f_mem_info:
if(line.find("MenTotal")==0):
men_info += line.strip()+","
elif(line.find("SwapTotal") == 0 ):
mem_info +=line.strip()
break
print("mem_info----{:s}".format(mem_info))
finally:
f_mem_info.close()
示例如下: kali Linux: 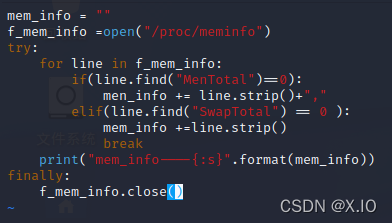 CentOS 7: 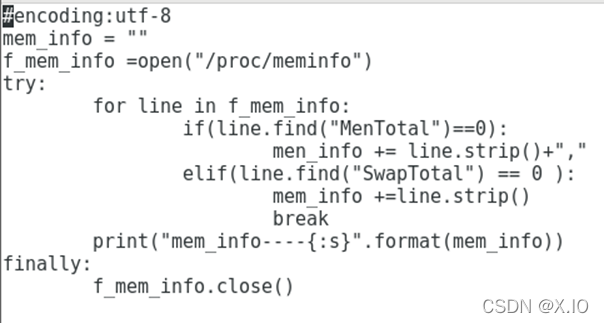 输出结果: 

4)查看-磁盘信息
#磁盘信息可以通过shell命令df -h查询
#encoding:utf-8
import subprocess
pipe = subprocess.Popen("df -h",stdout = subprocess.PIPE, shell=True)
disc_info = pipe.stdout.read()
print(disc_info)
示例如下: kali Linux:  CentOS 7:  输出结果: 
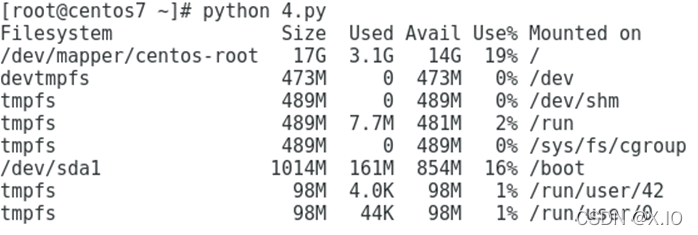
5)查看-网络信息
需要提前安装以下netifaces第三方库。
#使用netifaces模块可以获取本机IP、网关等信息。
#encoding:utf-8
def GetNetworkIP(): #获取本地网卡IP地址
import netifaces
#routingGateway = netifaces.gateways()['default'][netifaces.AF_INET][0] #网关
routingNicName = netifaces.gateways()['default'][netifaces.AF_INET][1] #网络适配器信息
for interface in netifaces.interfaces():
if interface == routingNicName:
#print(netifaces.ifaddresses(interface))
try:
routingIPAddr = netifaces.ifaddresses(interface)[netifaces.AF_INET][0]['addr'] #获取IP
except KeyError:
pass
#print("Routing IP Address:%s"% routingIPAddr)
return routingIPAddr
if __name__ =="__main__":
try:
print("Routing IP Address:",GetNetworkIP())
except:
print("Unable to get the address,there may not be installed netifaces module! command: pip install netifaces")
示例如下: kali Linux : 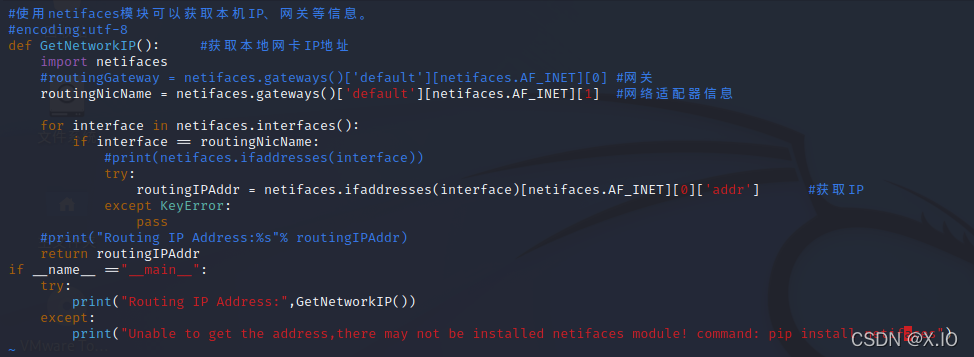
输出结果: 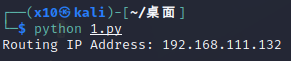
|