使用Servlet和JSP开发Java应用程序 ----错误处理
错误和异常处理
- 在 Web 应用程序中发生错误并引发异常
- 在服务通过发生错误消息和状态代码,并为客户提供冠以错误和异常的信息
- 状态代码是应用程序服务器发生给客户端的关于客户端请求是成功或失败的信息
- Http 状态代码用三位数表示
- 分为以下五个类别:
- 提示信息(1XX):表示请求已被接受,需要继续处理
- 成功(2XX):表示成功,请求被成功接收并处理
- 重定向(3XX):表示重定向,需要进一步的操作以完成请求处理,如跳转页面
- 客户端错误(4XX):表示客户端错误,它表示请求有错误导致无法完成请求处理,如语法错误
- 服务器错误(5XX):表示服务器错误
- 常见状态代码:200、301、304、400、404、410、500
配置错误页面
WEB-INF 下新建 web.xml 文件 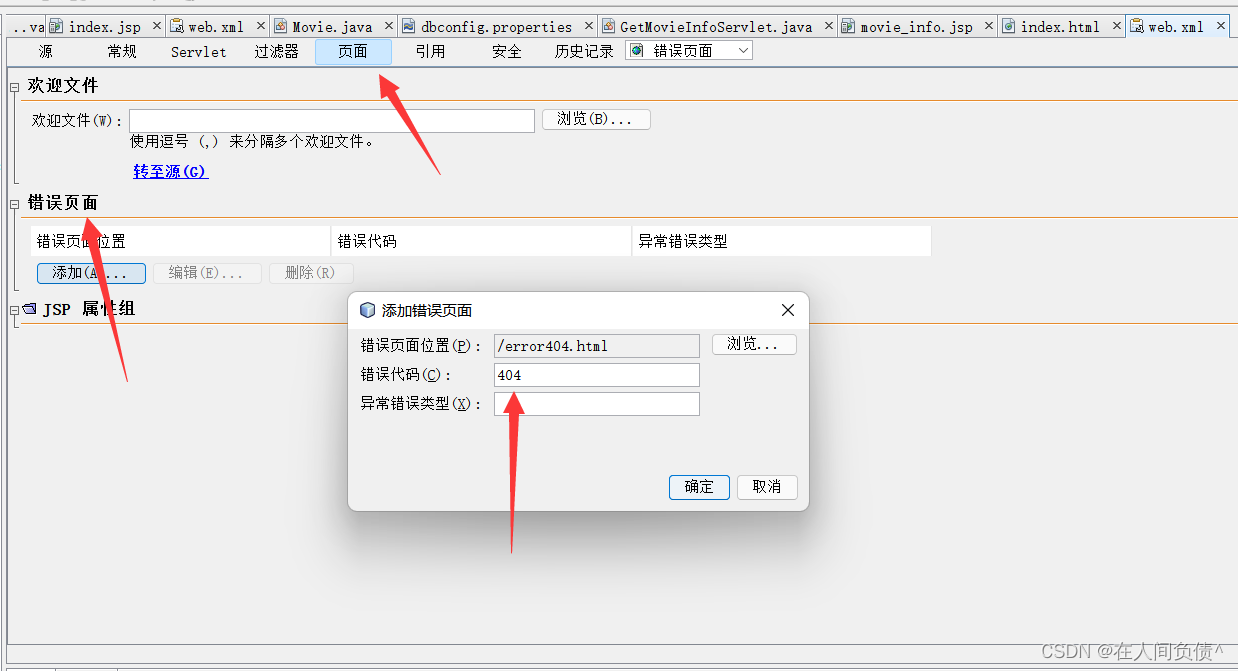
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="3.1" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd">
<session-config>
<session-timeout>
30
</session-timeout>
</session-config>
<error-page>
<error-code>404</error-code>
<location>/error404.html</location>
</error-page>
</web-app>
配置前的错误页面 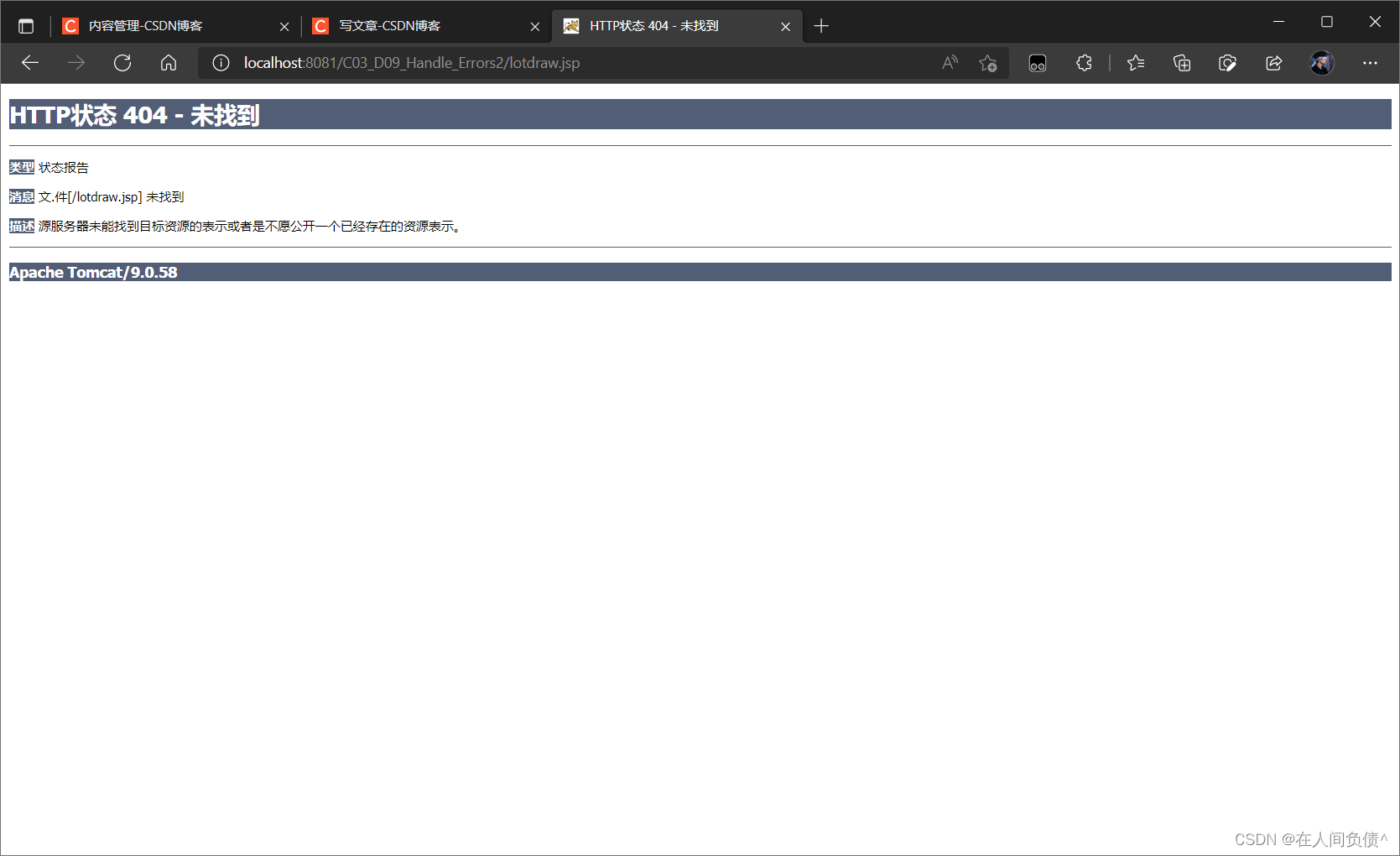
配置后的错误页面 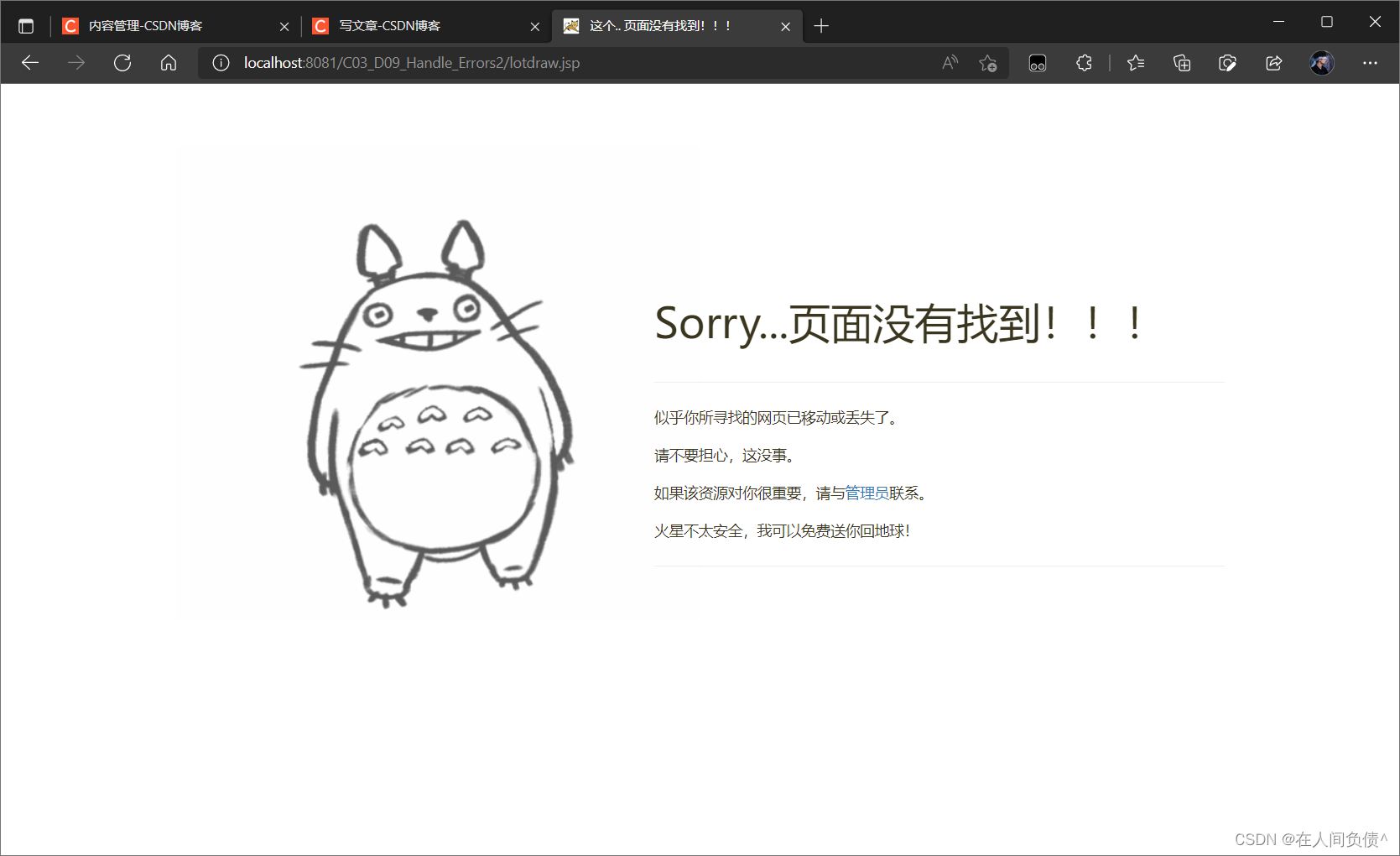
针对异常配置错误页面
算数异常 java.lang.ArithmeticException 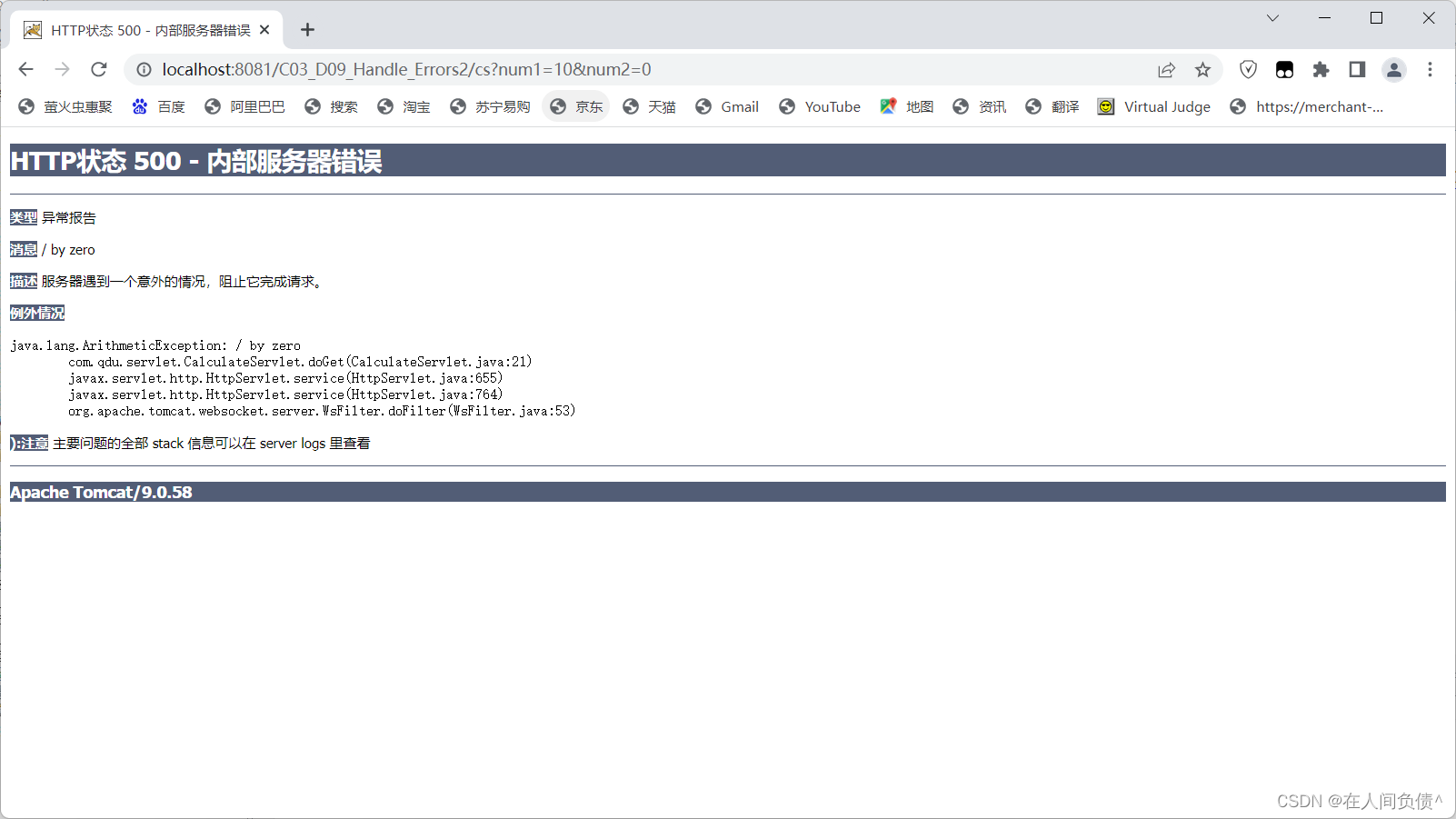 格式异常 java.lang.NumberFormatException 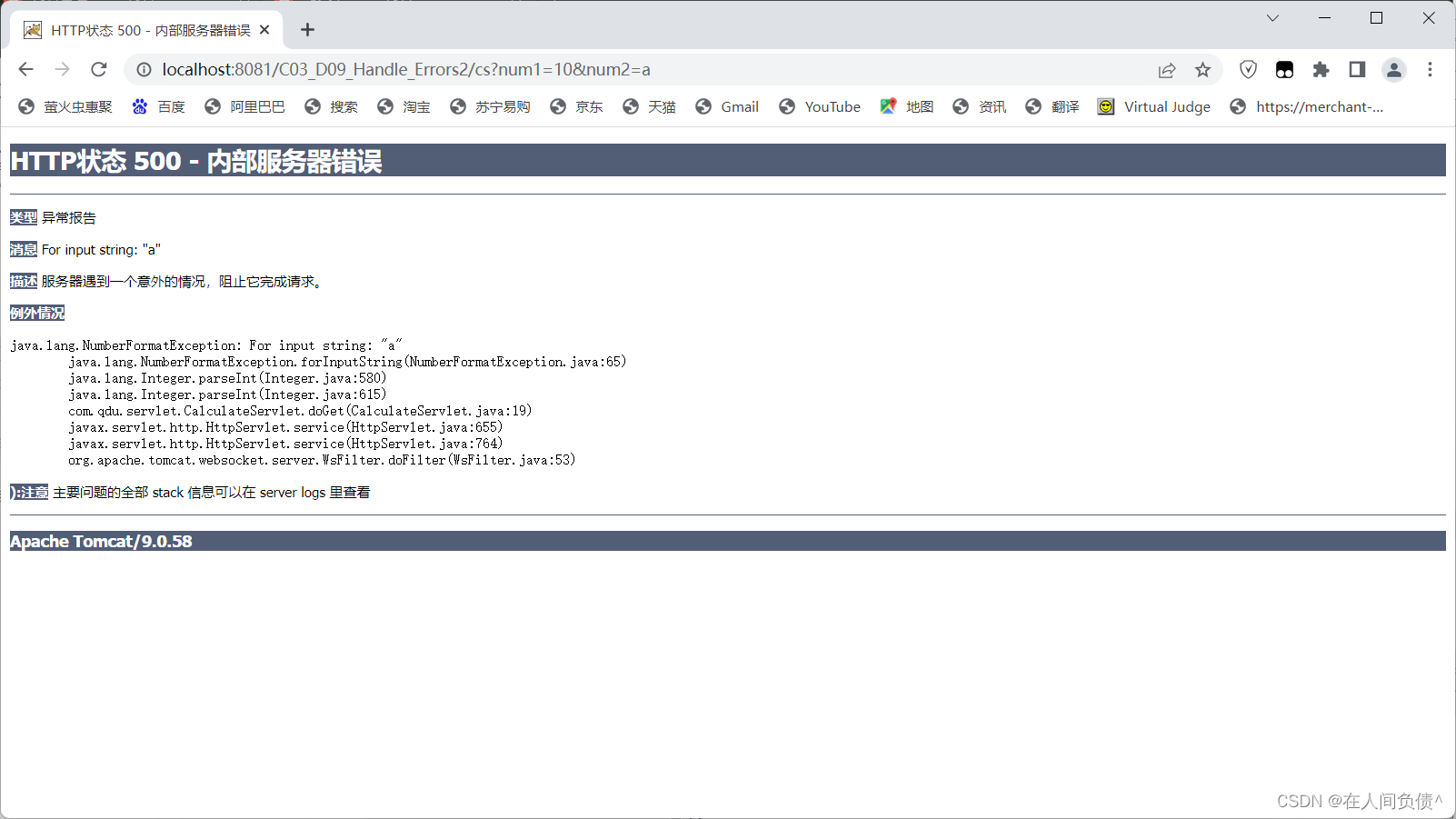
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="3.1" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd">
<session-config>
<session-timeout>
30
</session-timeout>
</session-config>
<error-page>
<error-code>404</error-code>
<location>/error404.html</location>
</error-page>
<error-page>
<exception-type>java.lang.ArithmeticException</exception-type>
<location>/error500.html</location>
</error-page>
<error-page>
<exception-type>java.lang.NumberFormatException</exception-type>
<location>/error500.html</location>
</error-page>
</web-app>
配置后的异常页面 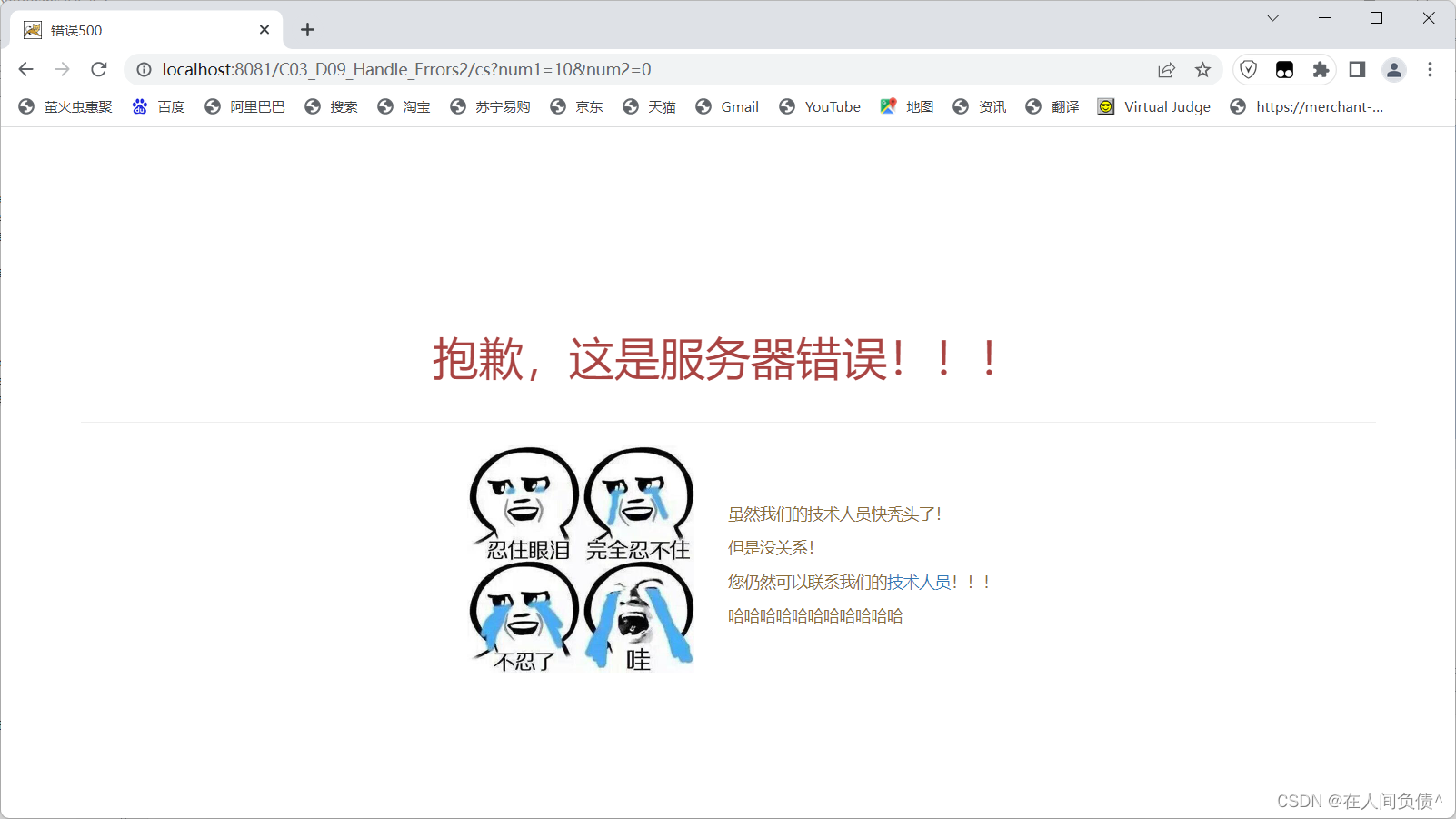
java.lang.Throwable 所有异常的基类 只要出现异常就会显示错误页面
<error-page>
<exception-type>java.lang.Throwable</exception-type>
<location>/error500.html</location>
</error-page>
使用 Servlet 配置错误页面
<error-page>
<exception-type>java.lang.Throwable</exception-type>
<location>/errorServlet</location>
</error-page>
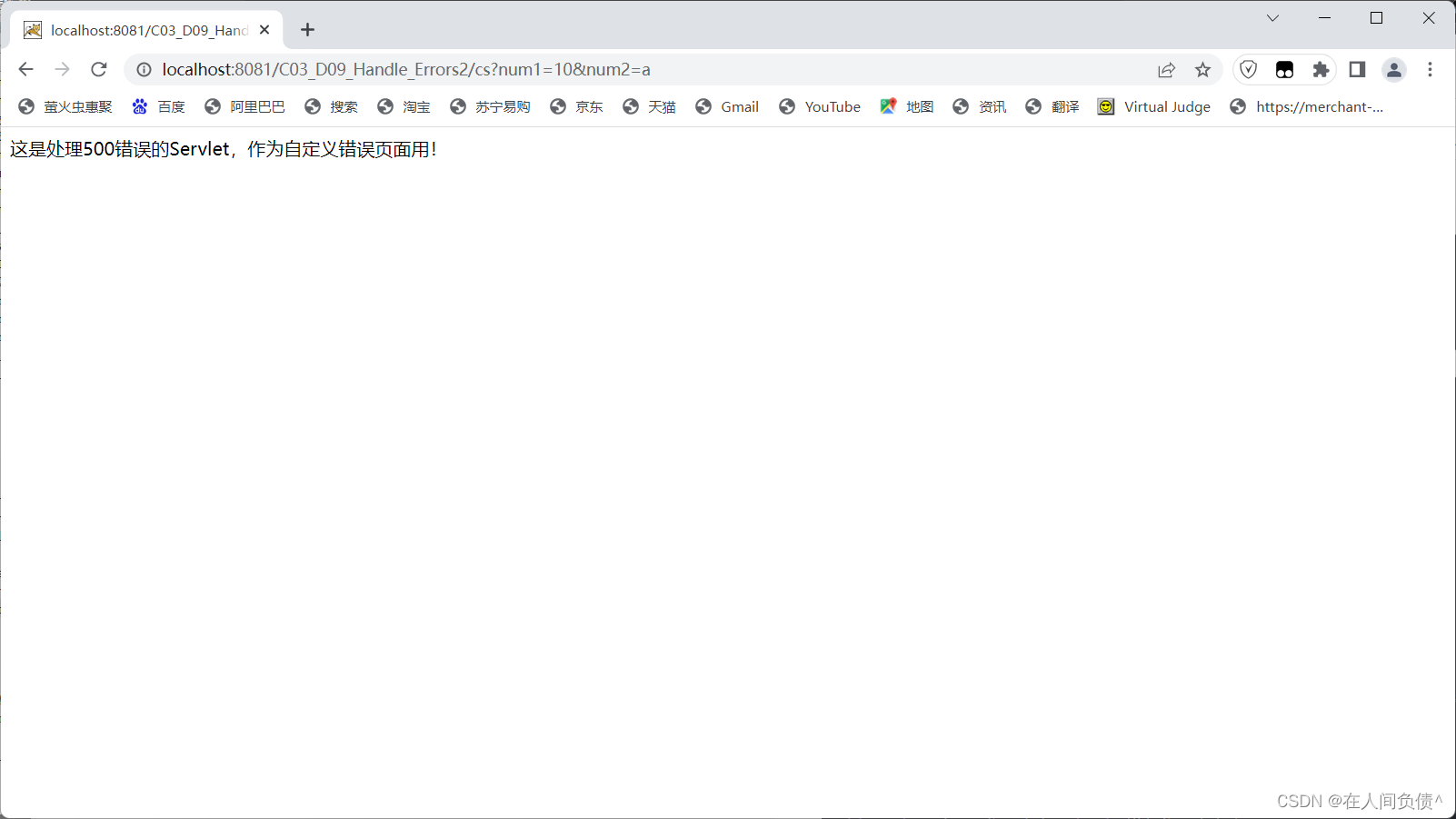
package com.qdu.servlet;
import java.io.IOException;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/errorServlet")
public class ErrorServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
ServletContext ctx = getServletContext();
ctx.log("....................................................................");
ctx.log("状态代码:" + req.getAttribute("javax.servlet.error.status_code"));
ctx.log("错误消息:" + req.getAttribute("javax.servlet.error.message"));
ctx.log("异常类型:" + req.getAttribute("javax.servlet.error.exception_type"));
ctx.log("....................................................................");
resp.setContentType("text/html;charset=utf-8");
resp.getWriter().println("这是处理500错误的Servlet,作为自定义错误页面用!");
}
}
控制台的输出 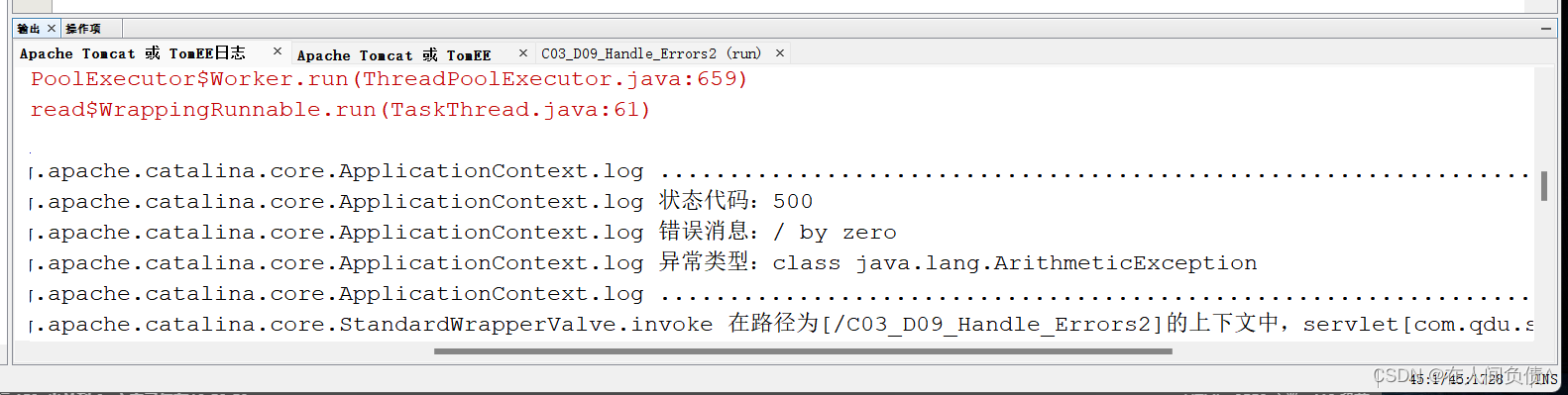
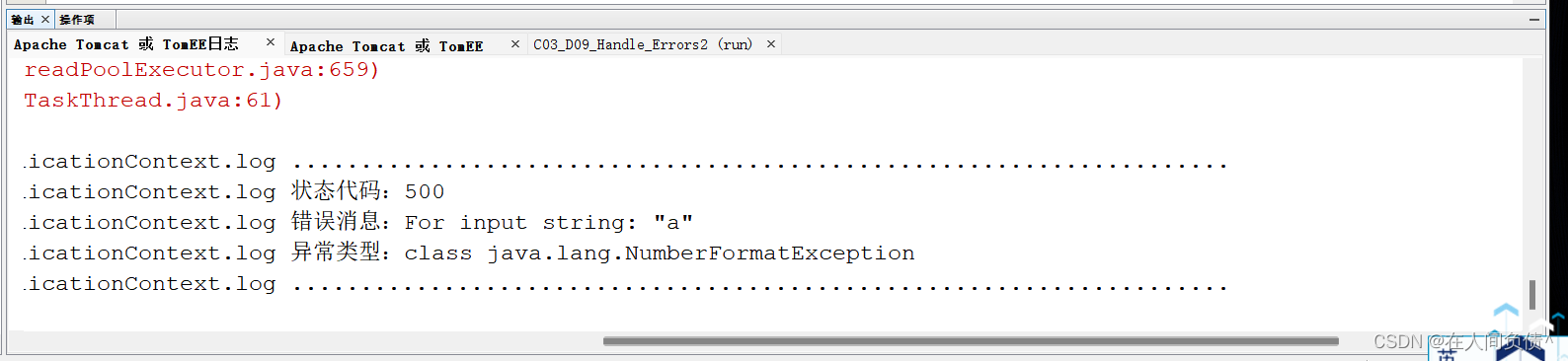
自己指定发送的状态代码
我们可以从客户端自己主动发送状态代码给服务器,得到相应的回应
setStatus() 发送非错误状态代码
setStatus() 方法(非错误状态码) 临时地 temporarily 302 临时重定向 永久地 permanently 301 永久重定向
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>百搜一下,你就知道</title>
<link rel="stylesheet" href="css/style.css"/>
</head>
<body>
<h1>百搜-搜天下</h1>
<form>
<input type="text">
<input type="submit" value="百搜一下">
</form>
<%
//如果想自己指定发送的状态代码,可以使用setStatus()方法(非错误状态码)或sendError()方法(错误状态码)
//永久地 permanently 301-永久重定向
//临时地 temporarily 302-临时重定向
response.sendRedirect("https://www.baidu.com");
//如果想要临时重定向到某个页面,可以使用302
//response.setStatus(302);
//如果想要永久重定向,可以使用301
response.setStatus(301);
response.setHeader("location", "https://www.baidu.com");
%>
</body>
</html>
sendError() 发送错误状态代码
可以调用 sendError() 自行指定发送给客户端的错误代码(4XX 或5XX ) 可以只指定发送的错误代码,也可同时指定发送的错误代码和一个消息
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>百搜一下,你就知道</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1>百搜搜-搜天下</h1>
<form>
<input type="text">
<input type="submit" value="百搜一下">
</form>
<%
//如果想自己指定发送的状态代码,可以使用setStatus()方法(非错误状态码)或sendError()方法(错误状态码)
//可以调用sendError()自行指定发送给客户端的错误代码(4XX或5XX)
//可以只指定发送的错误代码,也可同时指定发送的错误代码和一个消息
//1. 只指定发送的状态码
// response.sendError(404);
//2. 指定状态码的同时指定一个消息
// response.sendError(410,"请求的资源已经被永久删除了!!!");
//3. 也可使用HttpServletResponse接口中的常量来指定发送的状态码
response.sendError(HttpServletResponse.SC_GONE,"请求的资源已经被永久删除了!!!");
%>
</body>
</html>
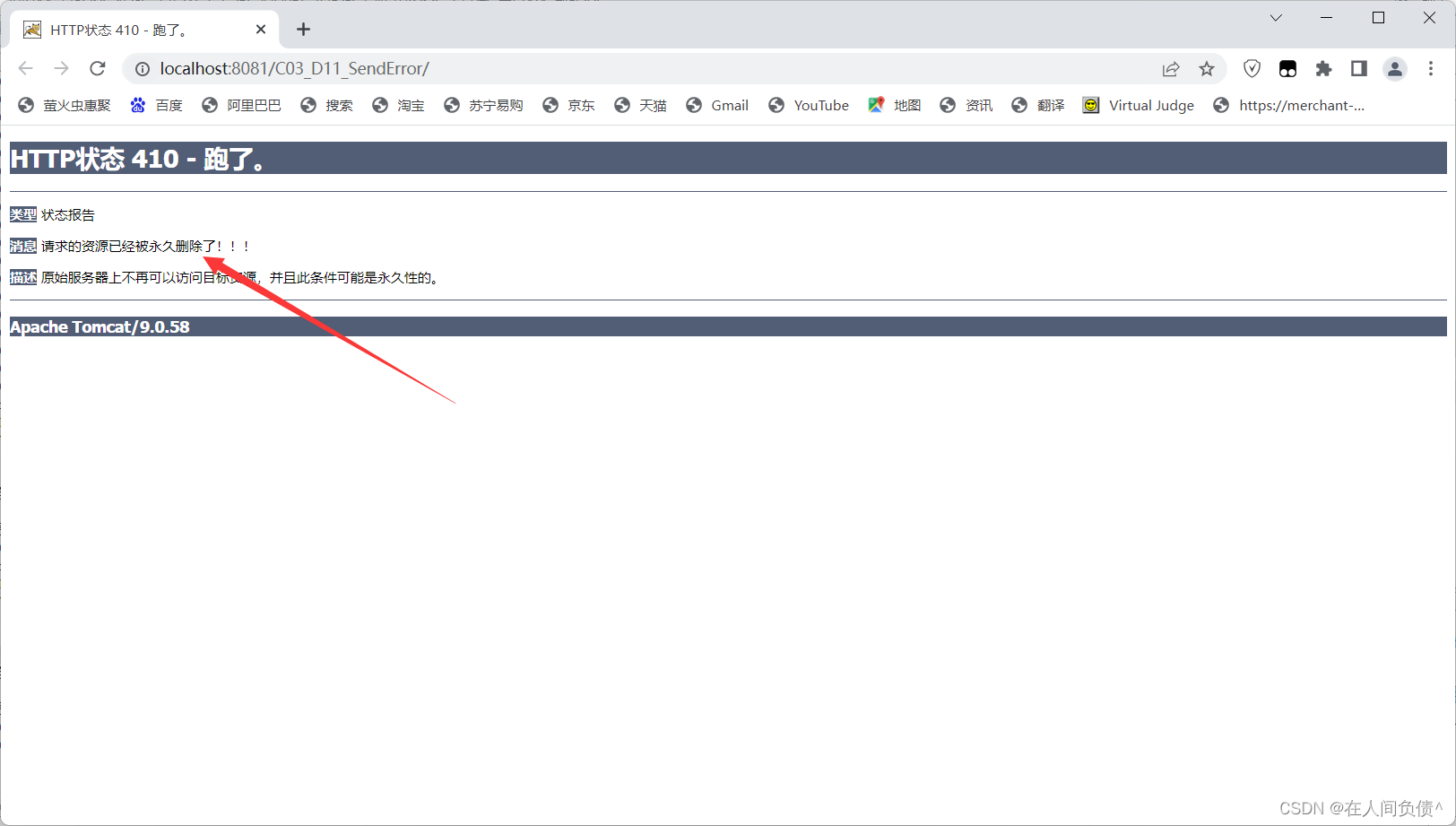
|