java从网络外链接下载文件并回传到其他网络服务器
场景
描述
从网络地址1下文件,然后将文件上传给网络地址2
举例
该地址为一个接收文件上传的接口
解决方案
将网络地址1上的文件下载到本地,然后再上传至网络地址2. 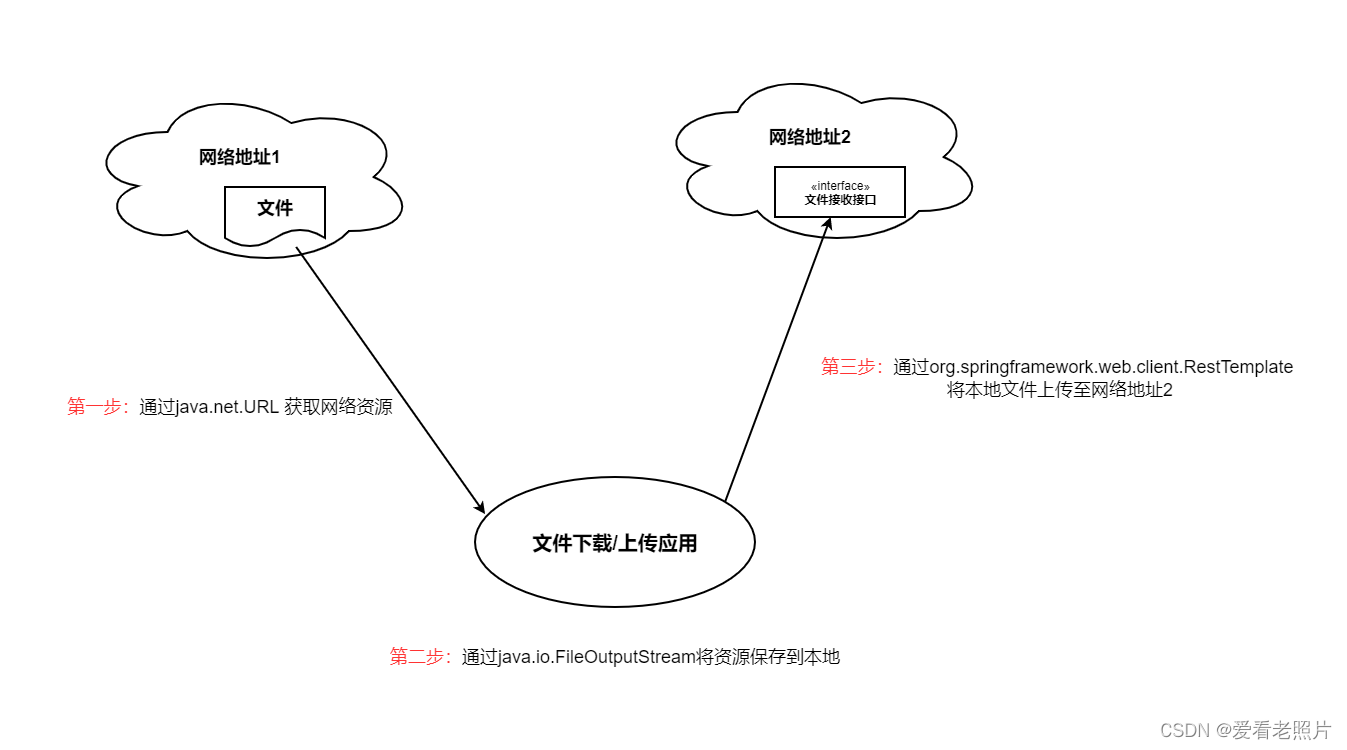
准备
package com.xl.test.logtest.controller;
import java.util.UUID;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
@RequestMapping("/file")
public class FileuploadController {
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) {
System.out.println("===================file.name======================"+file.getName());
System.out.println("===================file.size======================"+file.getSize());
return UUID.randomUUID().toString();
}
}
例子
package com.xl.test.json;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.net.URLConnection;
import org.apache.tomcat.util.http.fileupload.IOUtils;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
public class RemoteFileTest {
public static void main(String[] args) throws IOException {
String sourcePath = "https://cj.jj20.com/2020/down.html?picurl=/up/allimg/1111/0Q91Q50307/1PQ9150307-7.jpg";
String localTempPath = "E:\\localtempfile\\";
String destinationPath = "http://47.97.154.171:8080/file/upload";
URL url = new URL(sourcePath);
URLConnection connection = url.openConnection();
InputStream inputStream = connection.getInputStream();
File dir = new File(localTempPath);
if (!dir.exists()) {
dir.mkdirs();
}
File file = new File(localTempPath+"test.jpg");
FileOutputStream outputStream = new FileOutputStream(file);
IOUtils.copy(inputStream, outputStream);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.MULTIPART_FORM_DATA);
MultiValueMap<String, Object> body = new LinkedMultiValueMap<String, Object>();
body.add("file", new FileSystemResource(file));
HttpEntity<MultiValueMap<String, Object>> requestEntity = new HttpEntity<MultiValueMap<String, Object>>(body, headers);
RestTemplate template = getTemplate();
ResponseEntity<String> response = template.postForEntity(destinationPath, requestEntity, String.class);
String data = response.getBody();
System.out.println("目标地址(上传接口)返回的数据data = "+data);
inputStream.close();
outputStream.close();
}
private static RestTemplate getTemplate() {
SimpleClientHttpRequestFactory factory = new SimpleClientHttpRequestFactory();
factory.setReadTimeout(180000);
factory.setConnectTimeout(5000);
RestTemplate template = new RestTemplate(factory);
return template;
}
}
说明,如果是springboot项目
- 生成 RestTemplate 实例使用@Bean注解方式,如下:
@Bean
public RestTemplate restTemplate(ClientHttpRequestFactory factory) {
return new RestTemplate(factory);
}
@Bean
public ClientHttpRequestFactory simpleClientHttpRequestFactory() {
SimpleClientHttpRequestFactory factory = new SimpleClientHttpRequestFactory();
factory.setReadTimeout(180000);
factory.setConnectTimeout(5000);
return factory;
}
- 使用RestTemplate 实例,直接使用@Autowired注解,如
@Autowired
private RestTemplate template;
测试
直接运行main方法即可 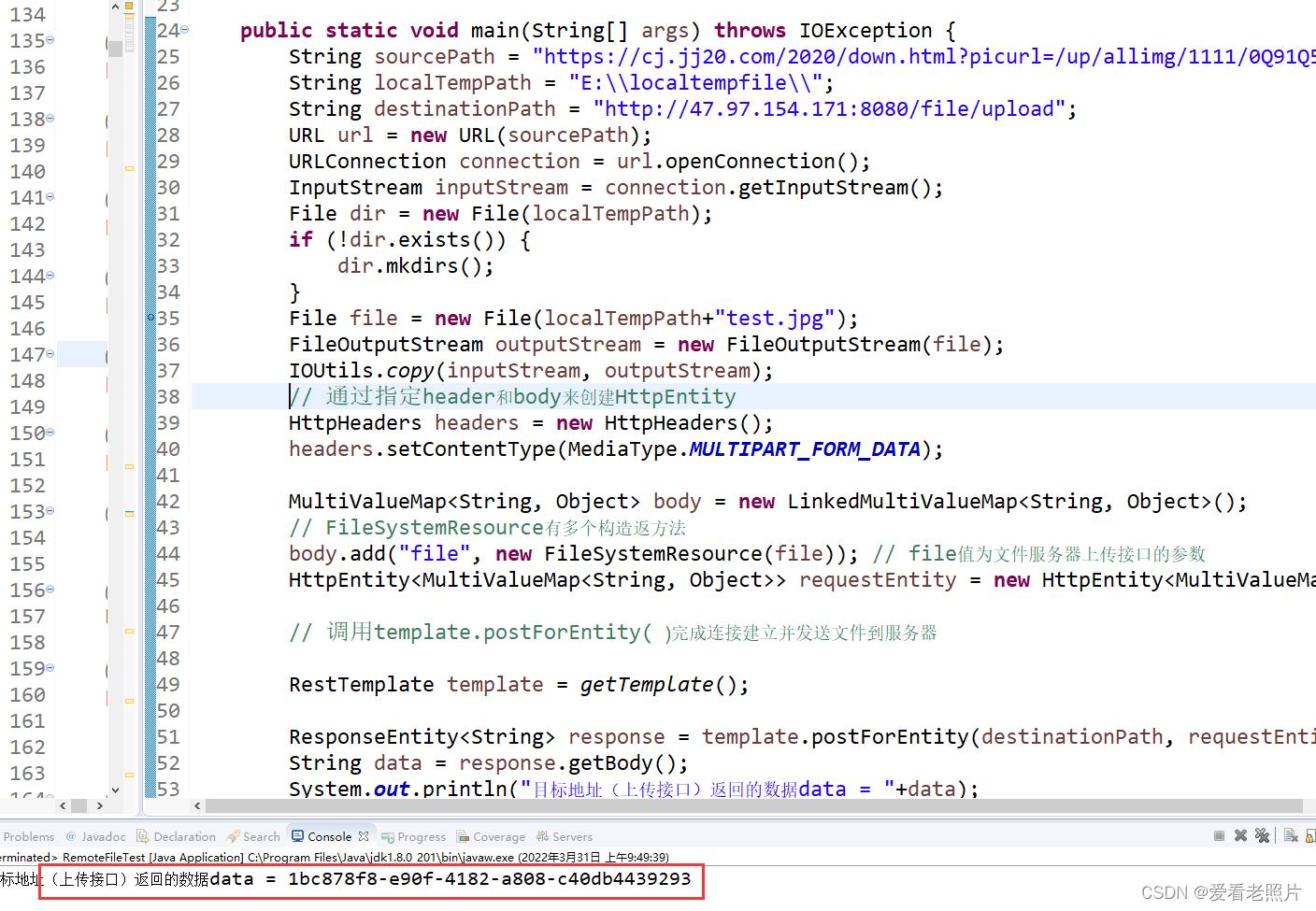 在网络地址2查看文件接收情况 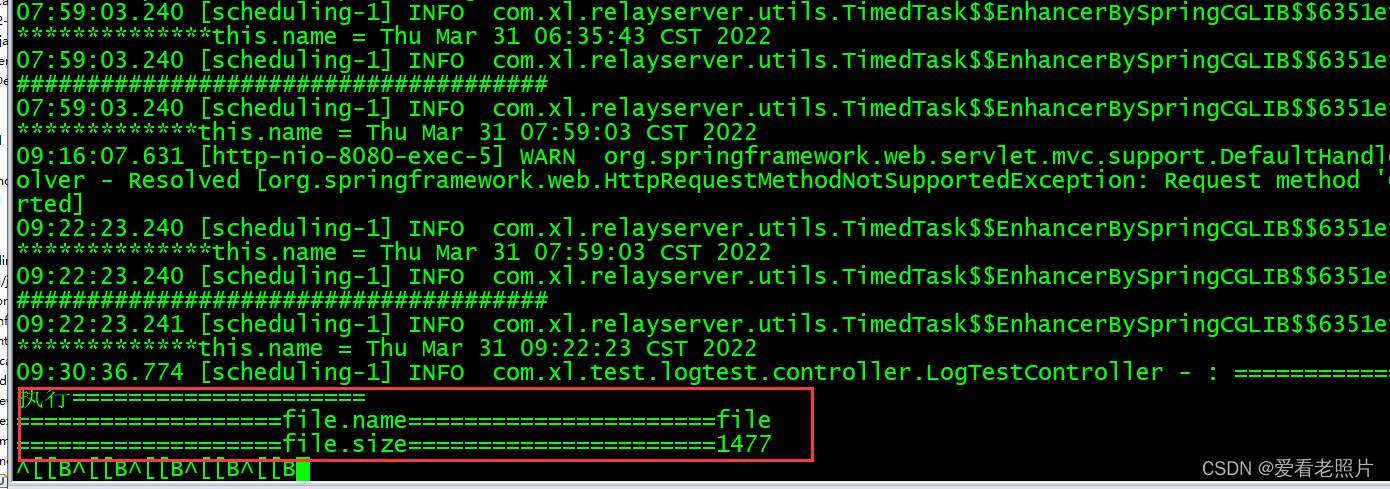
其他更细粒度实现的文件上传至远程(网络)服务器例子
java上传文件到远程服务器(一)—HttpURLConnection方式
java上传文件到远程服务器(二)—HttpClient方式
|