一、前言
在linux的新内核中,可以根据下面文件操作集中的结构体看出,旧内核中ioctl已经被unlocked_ioctl所替代,在编写驱动时,如果驱动需要与应用传输数组或都是结构体,很多人就不知所措,下面我用两个例子来实现应用层与驱动层通过ioctl传输数据。
struct file_operations {
struct module *owner;
loff_t (*llseek) (struct file *, loff_t, int);
ssize_t (*read) (struct file *, char __user *, size_t, loff_t *);
ssize_t (*write) (struct file *, const char __user *, size_t, loff_t *);
ssize_t (*aio_read) (struct kiocb *, const struct iovec *, unsigned long, loff_t);
ssize_t (*aio_write) (struct kiocb *, const struct iovec *, unsigned long, loff_t);
int (*readdir) (struct file *, void *, filldir_t);
unsigned int (*poll) (struct file *, struct poll_table_struct *);
long (*unlocked_ioctl) (struct file *, unsigned int, unsigned long);
long (*compat_ioctl) (struct file *, unsigned int, unsigned long);
int (*mmap) (struct file *, struct vm_area_struct *);
int (*open) (struct inode *, struct file *);
int (*flush) (struct file *, fl_owner_t id);
int (*release) (struct inode *, struct file *);
int (*fsync) (struct file *, loff_t, loff_t, int datasync);
int (*aio_fsync) (struct kiocb *, int datasync);
int (*fasync) (int, struct file *, int);
int (*lock) (struct file *, int, struct file_lock *);
ssize_t (*sendpage) (struct file *, struct page *, int, size_t, loff_t *, int);
unsigned long (*get_unmapped_area)(struct file *, unsigned long, unsigned long, unsigned long, unsigned long);
int (*check_flags)(int);
int (*flock) (struct file *, int, struct file_lock *);
ssize_t (*splice_write)(struct pipe_inode_info *, struct file *, loff_t *, size_t, unsigned int);
ssize_t (*splice_read)(struct file *, loff_t *, struct pipe_inode_info *, size_t, unsigned int);
int (*setlease)(struct file *, long, struct file_lock **);
long (*fallocate)(struct file *file, int mode, loff_t offset,
loff_t len);
};
二、应用层向驱动层传输结构体
2.1 应用层向驱动层传递数据并控制硬件
本次设计为应用层向驱动层传递结构体,并通过解析数据,控制开发板LED灯,设计的结构体如下:
typedef struct {
unsigned char ltype;
unsigned int state;
unsigned char name[20];
unsigned int *prv;
}fpga_opt_t;
直接上应用层代码
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <sys/ioctl.h>
typedef struct {
unsigned char ltype;
unsigned int state;
unsigned char name[20];
unsigned int *prv;
}fpga_opt_t;
#define GEC6818_struct_R _IOR('S', 1, fpga_opt_t)
#define GEC6818_struct_W _IOW('S', 2, fpga_opt_t)
#define LED_FILE "/dev/gec6818_leds"
int main(void)
{
int led_fd, len;
fpga_opt_t temp = {'L', 1, "Light test", NULL};
led_fd = open(LED_FILE, O_RDWR);
if(led_fd == -1)
{
printf("open failure\n");
}
while(1)
{
temp.state = 1;
sleep(2);
len = ioctl(led_fd, GEC6818_struct_R, &temp);
temp.state = 0;
sleep(2);
len = ioctl(led_fd, GEC6818_struct_R, &temp);
}
close(led_fd);
}
直接上驱动代码
#include <linux/module.h>
#include <linux/init.h>
#include <linux/kernel.h>
#include <linux/cdev.h>
#include <linux/fs.h>
#include <linux/uaccess.h>
#include <linux/device.h>
#include <linux/ioport.h>
#include <linux/io.h>
#include <linux/gpio.h>
#include <cfg_type.h>
#include <linux/miscdevice.h>
#include <linux/ioctl.h>
typedef struct {
unsigned char ltype;
unsigned int state;
unsigned char name[20];
unsigned int *prv;
}fpga_opt_t;
#define GEC6818_struct_R _IOR('S', 1, fpga_opt_t)
#define GEC6818_struct_W _IOW('S', 2, fpga_opt_t)
fpga_opt_t tmp = {0};
fpga_opt_t *ptmp = &tmp;
static int gec6818_led_open(struct inode *inode, struct file *file)
{
gpio_direction_output(PAD_GPIO_E+13, 1);
printk("gec6818_led_open \n");
return 0;
}
static ssize_t gec6818_led_read(struct file *file, char __user *buf, size_t len, loff_t * off)
{
int klen , rt;
char kbuf[16] = "this is kernel";
rt = copy_to_user(buf, kbuf , len);
if(rt)
{
return -EINVAL;
}
printk("gec6818_led_read \n");
return 0;
}
static ssize_t gec6818_led_write(struct file *file , const char __user *buf, size_t len, loff_t * off)
{
return 0;
}
int kernel_strcpy(const char *strSrc, char *strDestCopy)
{
int i, len = 0;
if ((NULL==strSrc) || (NULL==strDestCopy))
return -1;
while(*strSrc != 0)
{
*strDestCopy = *strSrc;
strDestCopy++;
strSrc++;
}
return 0;
}
static long gec6818_led_unlocked_ioctl(struct file *filp, unsigned int cmd, unsigned long args)
{
int rt;
switch(cmd)
{
case GEC6818_struct_R:
copy_from_user((unsigned char *)ptmp,(unsigned char *)args,sizeof(fpga_opt_t));
printk("ptmp->ltype:%c, ptmp->state:%d,ptmp->name:%s, ptmp->prv:%p\n",
ptmp->ltype, ptmp->state, ptmp->name, ptmp->prv);
if(ptmp->ltype == 'L')
{
if(ptmp->state == 1)
gpio_set_value(PAD_GPIO_E+13, 0);
if(ptmp->state == 0)
gpio_set_value(PAD_GPIO_E+13, 1);
}
break;
case GEC6818_struct_W:
ptmp->ltype = 'B';
ptmp->state++;
kernel_strcpy( "this is beep state", ptmp->name);
printk("ptmp->name:%s\n", ptmp->name);
ptmp->prv = NULL;
copy_to_user((unsigned char *)args, (unsigned char *)ptmp , sizeof (fpga_opt_t) );
break;
default:
break;
}
return rt;
}
static int gec6818_led_release (struct inode *inode, struct file *file)
{
printk("gec6818_led_release\n");
return 0;
}
static struct file_operations gec6818_led_ops =
{
.owner = THIS_MODULE,
.open = gec6818_led_open,
.read = gec6818_led_read,
.unlocked_ioctl=gec6818_led_unlocked_ioctl,
.write = gec6818_led_write,
.release = gec6818_led_release,
};
static struct miscdevice gec6818_led_miscdev = {
.minor = MISC_DYNAMIC_MINOR,
.name = "gec6818_leds",
.fops = &gec6818_led_ops,
};
static int __init gec6818_led_init(void)
{
struct resource *gpioes;
int rt;
rt = misc_register(&gec6818_led_miscdev);
if (rt) {
printk("misc_register_fail\n");
goto misc_register_fail;
}
gpio_free(PAD_GPIO_E+13);
rt = gpio_request(PAD_GPIO_E+13, "gpioe13");
if(rt)
{
printk("gpio_request_fail\n");
goto gpio_request_fail;
}
printk("gec6818_led_init\n");
return 0;
gpio_request_fail:
gpio_free(PAD_GPIO_E+13);
misc_register_fail:
misc_deregister(&gec6818_led_miscdev);
return rt;
}
static void __exit gec6818_led_exit(void)
{
gpio_free(PAD_GPIO_E+13);
misc_deregister(&gec6818_led_miscdev);
printk("gec6818_led_exit\n");
}
module_init(gec6818_led_init);
module_exit(gec6818_led_exit);
MODULE_AUTHOR("pqrs8889@163.com");
MODULE_DESCRIPTION("gec6818_led driver");
MODULE_LICENSE("GPL");
2.2 实验效果
CRT打印信息 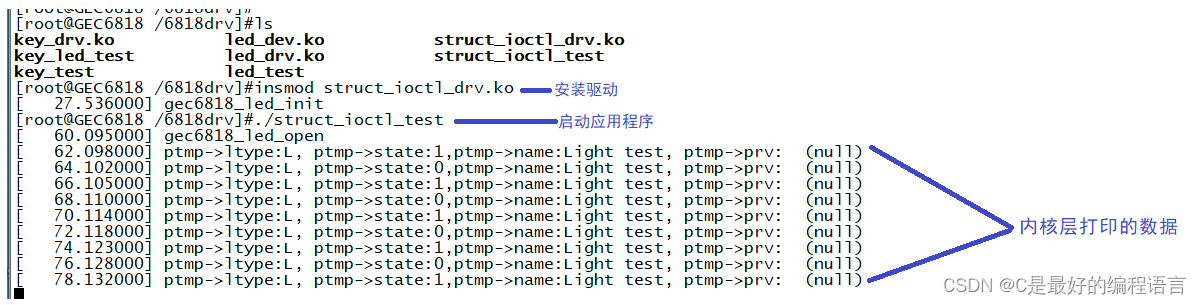 硬件显示效果,硬件的亮与灭,我觉得我不会在CSDN中搞动图,等我搞会了,再搞动图了。
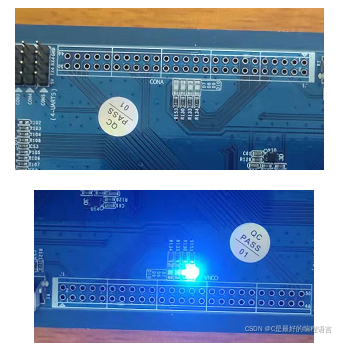
三、驱动层向应用层传递结构体
3.1驱动层向应用层传递结构体
本次设计为驱动层向应用传递结构体,应用层将收到的数据进行打印出来,结构体如下,好吧,其实就是那个灯的结构体,就懒得改了,将就用哈。
typedef struct {
unsigned char ltype;
unsigned int state;
unsigned char name[20];
unsigned int *prv;
}fpga_opt_t;
直接上应用层代码
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <sys/ioctl.h>
typedef struct {
unsigned char ltype;
unsigned int state;
unsigned char name[20];
unsigned int *prv;
}fpga_opt_t;
#define GEC6818_struct_R _IOR('S', 1, fpga_opt_t)
#define GEC6818_struct_W _IOW('S', 2, fpga_opt_t)
#define LED_FILE "/dev/gec6818_leds"
int main(void)
{
int led_fd, len;
fpga_opt_t temp = {0};
led_fd = open(LED_FILE, O_RDWR);
if(led_fd == -1)
{
printf("open failure\n");
}
while(1)
{
len = ioctl(led_fd, GEC6818_struct_W, &temp);
printf("temp.ltype:%c, temp.state:%d,temp.name:%s, temp.prv:%p\n",
temp.ltype, temp.state, temp.name, temp.prv);
sleep(2);
}
close(led_fd);
}
这里的驱动层代码,将参考2.1中的驱动代码!这里的驱动层代码,将参考2.1中的驱动代码!这里的驱动层代码,将参考2.1中的驱动代码!
3.1 实验效果
由于这里只是获取数据,到时大家可以灵活去上传自己想要上传的数据,比如驱动温度、温度,X轴等值。这里就不搞了。
驱动中上报数据
case GEC6818_struct_W:
ptmp->ltype = 'B';
ptmp->state++;
kernel_strcpy( "this is beep state", ptmp->name);
printk("ptmp->name:%s\n", ptmp->name);
ptmp->prv = NULL;
copy_to_user((unsigned char *)args, (unsigned char *)ptmp , sizeof (fpga_opt_t) );
break;
实验效果及分析 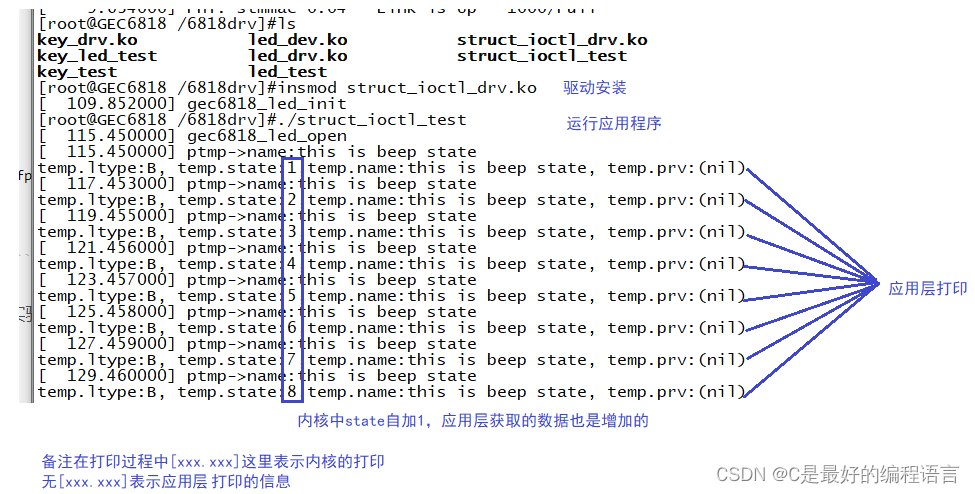
|