基于Linux、Win、OpenCV、树莓派、OpenMV、MicroPython等大部分开发环境的网络摄像头图像输出(将本地摄像头转为网络,可进行人脸检测)
开发环境中得有Python环境 Python环境自带Flask库 另外需要采集内置摄像头图像 这里用的为OpenCV库实现 同样也可以用picamera等可以调用摄像头的库 或者直接从底层调用摄像头驱动
开发环境中可以配置多进程开机自动运行 使其在调用的同时也可以进行其他工作 在代码上可以增加控制功能 包括系统开关等 可扩展性高
以Linux环境为例 配置开机自动运行的脚本为:
[Desktop Entry]
Name=dotnet
Comment=dotnet Program
Exec=lxterminal -e sudo python3 /home/pi/Desktop/Test/Track_All_1.0.py
Terminal=True
MultipleArgs=false
Type=Application
Categories=Application;Development;
StartupNotify=true
首先建立服务器,同时用OpenCV调用本地摄像头采集图像 并转为比特格式
代码如下:
from flask import Flask,Response
import cv2
local_post = 1212
app = Flask(__name__)
cap = cv2.VideoCapture(0)
def send_img():
while True:
ok, faceImg = cap.read()
if ok is False:
break
image = cv2.imencode('.jpg', faceImg)[1].tobytes()
yield (b'--frame\r\n'
b'Content-Type: image/jpeg\r\n\r\n' + image + b'\r\n')
return
@app.route('/video_feed')
def video_feed():
return Response(send_img(), mimetype='multipart/x-mixed-replace; boundary=frame')
@app.route('/')
def hello():
return 'Hello World!'
if __name__ == "__main__":
app.run(host='0.0.0.0', port=local_post)
服务器建立好后 IP地址+端口1212+video_feed地址下则为图像视频流 如192.168.1.1:1212/video_feed/
若需要进行网络控制 则新建一个HTLM文件 如index.html
输入HTLM语言程序:
<html>
<head>
<title>局域网视频推流及控制系统</title>
</head>
<body>
<h1>局域网视频推流及控制系统</h1>
<form action="/" method="post" style="float:left">
<p>
<input type="submit" style="font-size:60px" name="auto" value="自动模式">
<input type="submit" style="font-size:60px" name="manu" value="手动模式">
</p>
<p>
<input type="submit" style="font-size:60px" name="reset" value="复位导轨">
<input type="submit" style="font-size:60px" name="set" value="设置点位">
</p>
<p>
<input type="submit" style="font-size:60px" name="left" value="左移导轨">
<input type="submit" style="font-size:60px" name="right" value="右移导轨">
</p>
<p>
<input type="submit" style="font-size:60px" name="go_in" value="继续程序">
<input type="submit" style="font-size:60px" name="go_out" value="暂停程序">
</p>
<p>
<input type="submit" style="font-size:60px" name="stop" value="停止导轨">
<input type="submit" style="font-size:60px" name="quit" value="全部退出">
</p>
</form>
<img src="{{ url_for('video_feed') }}" height="520" style="float:left">
</body>
</html>
打开IP地址+端口号即可看到实时图像并进行网络控制
用多线程函数加入人脸识别及网络控制的改进效果即:
import cv2
from flask import Flask, render_template, Response, request
import threading
import socket
local_ip = str(socket.gethostbyname(socket.gethostname()))
local_post = 1212
app = Flask(__name__)
cap = cv2.VideoCapture(0)
classifier = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
@app.route('/', methods=['GET', 'POST'])
def index():
global command_str
command_str = None
if request.method == 'POST':
c0 = str(request.form.get('change'))
c1 = str(request.form.get('quit'))
c2 = str(request.form.get('left'))
c3 = str(request.form.get('right'))
c4 = str(request.form.get('stop'))
c5 = str(request.form.get('reset'))
c6 = str(request.form.get('set'))
print(c0,c1,c2,c3,c4,c5,c6)
for i in [c0,c1,c2,c3,c4,c5,c6]:
if i != "None":
command_str = i
break
print(command_str)
return render_template('index.html')
def track():
global faceImg
global q
global command_str
command_str = None
q = 0
while True:
ok, faceImg = cap.read()
faceImg = cv2.flip(faceImg,1)
if ok is False:
print('无法读取到摄像头!')
break
gray = cv2.cvtColor(faceImg,cv2.COLOR_BGR2GRAY)
faceRects = classifier.detectMultiScale(gray,scaleFactor=1.2,minNeighbors=3,minSize=(32, 32))
if len(faceRects):
for faceRect in faceRects:
x,y,w,h = faceRect
cv2.rectangle(faceImg,(x, y), (x + w, y + h), (0,255,0), 2)
cv2.imshow("http://"+local_ip+":"+str(local_post)+"/ (img: video_feed)",faceImg)
if command_str == "复位":
print("Reset")
elif command_str == "标定":
print("Set")
elif command_str == "切换":
print("Change")
elif command_str == "左移":
print("Left")
elif command_str == "右移":
print("Right")
elif command_str == "停止":
print("Stop")
else:
command_str = command_str
if cv2.waitKey(10) == 27 or command_str == "退出":
command_str = None
print("Quit")
break
command_str = None
cap.release()
cv2.destroyAllWindows()
q = 1
def send_img():
global faceImg
global q
q = 0
while True:
image = cv2.imencode('.jpg', faceImg)[1].tobytes()
yield (b'--frame\r\n'
b'Content-Type: image/jpeg\r\n\r\n' + image + b'\r\n')
if q==1:
break
return
@app.route('/video_feed')
def video_feed():
return Response(send_img(), mimetype='multipart/x-mixed-replace; boundary=frame')
def start_server():
app.run(host='0.0.0.0', port=local_post)
thread_img = threading.Thread(target=track)
thread_img.setDaemon(True)
thread_img.start()
thread_send = threading.Thread(target=start_server)
thread_send.setDaemon(True)
thread_send.start()
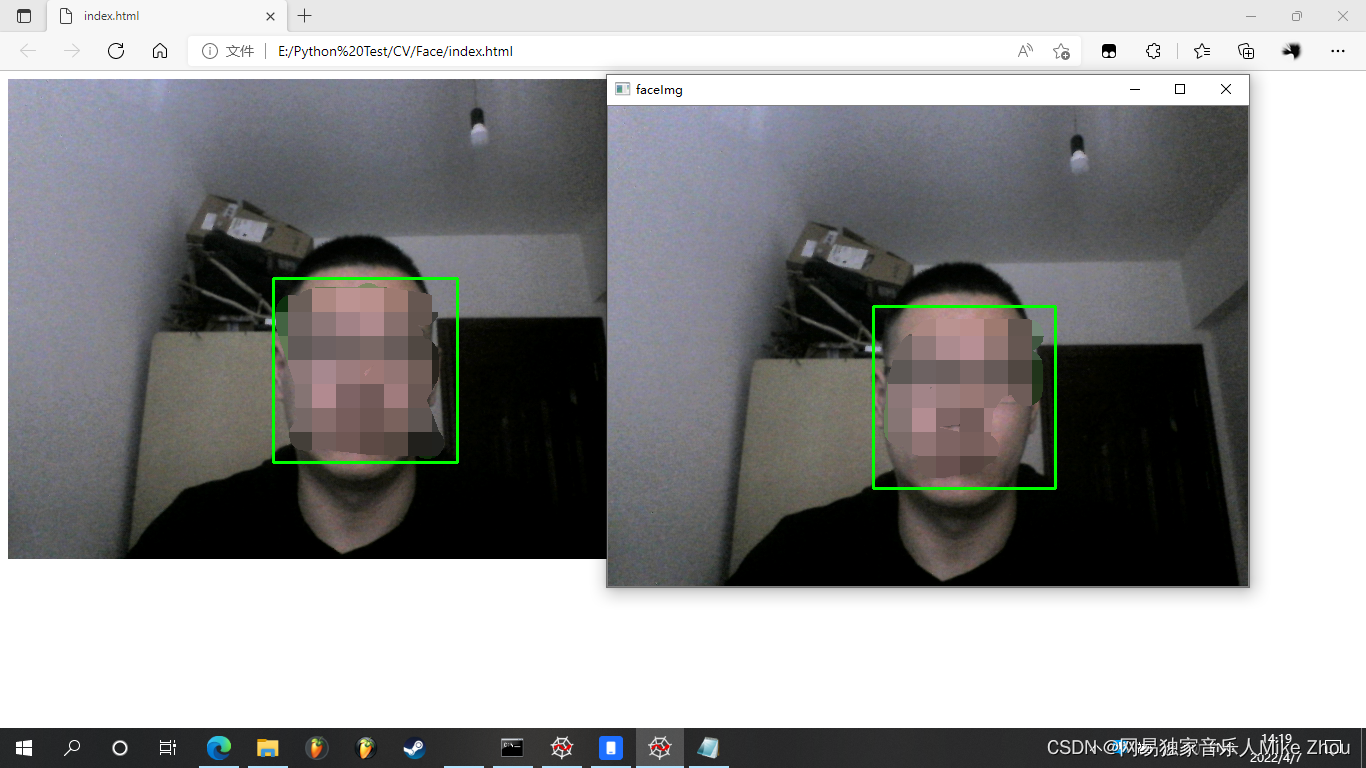
|