提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档
前言
用shell编程来实现对系统用户的增删改查 目录结构 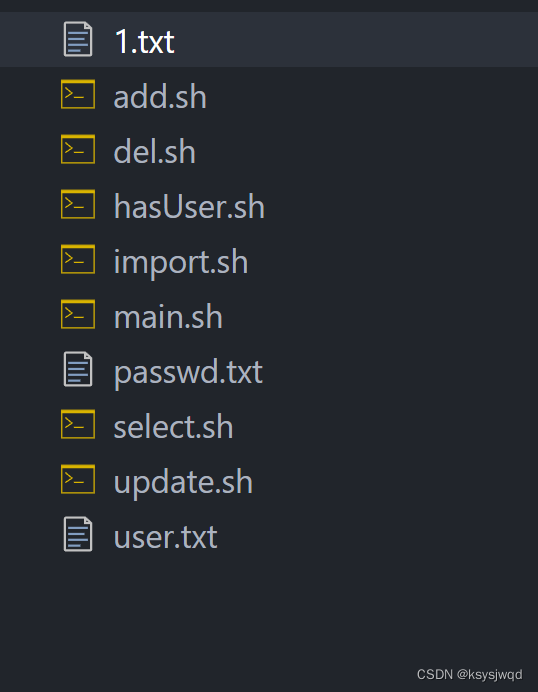 目录结构说明 1.txt文件是用来存储错误的命令或者正确的命令的,用来保持控制台的美观 add.sh是用来添加用户的,这里可以简单的添加,也可以复杂的添加,可以指定用户组家目录,使用的shell和描述信息 del.sh是用来删除用户的 hasUser.sh是用来判断用户是否存在的 import.sh是用来批量导入用户的 passwd.sh是用来存储用户的密码的,在进行其他操作时,用户的相关值也会发生改变 select.sh是用来显示用户的 update.sh是用来修改用户的信息的,可以修改密码或者其他的信息 user.txt是用来批量导入用户的,在进行其他操作时,用户的相关值也会发生改变
一、代码展示
main文件
#!/bin/bash
function isEmpty(){
string=`sed -n 'p' user.txt`
if [ -z "${string}" ]
then
echo "还没有用户,请添加用户"
continue
fi
}
if [ $(whoami) = "root" ]
then
while true
do
echo "请输入数字:1为增加用户,2为删除用户,3为修改用户信息,4为查询所有用户,5为批量导入数据,6为退出bash"
read num
case ${num} in
1)
read -p "请输入要增加的用户名:" username
bash hasUser.sh ${username}
if [ $? -eq 0 ]
then
echo "用户名已经存在!"
continue
fi
read -p "请输入用户名密码:" password
read -n 1 -p "是否要指定其他用户信息y/n:" flag
bash add.sh ${username} ${password} ${flag}
;;
2)
isEmpty
read -p "请输入要删除的用户:" username
bash hasUser.sh ${username}
if [ $? -eq 1 ]
then
echo "用户不存在!"
continue
fi
bash del.sh ${username}
;;
3)
isEmpty
read -p "请输入要修改的用户名:" username
bash hasUser.sh ${username}
if [ $? -eq 1 ]
then
echo "用户不存在!"
continue
fi
read -n 1 -p "是要修改用户密码还是其他用户信息,1为修改密码,2为修改用户其他信息" flag
bash update.sh ${username} ${flag}
;;
4)
bash select.sh
;;
5)
bash import.sh
;;
6)
exit
;;
*)
echo "请输入正确的数字"
;;
esac
done
else
echo "需要切换到root用户,请输入root用户密码"
su root
fi
1.添加用户
#!/bin/bash
function add()
{
if [ $3 = n ]
then
homeDir="/home/"${1}
shell="/bin/bash"
echo
useradd $1
elif [ $3 = y ]
then
echo
read -p "请输入所属组名:" group
read -p "请输入家目录:" homeDir
read -p "请输入用户使用的shell:" shell
read -p "请输入用户描述信息:" message
string=$(sed -n "/^${group}/p" /etc/group)
if [ -z ${string} ]
then
groupadd ${group}
fi
echo
useradd -d ${homeDir} -s ${shell} -g ${group} -c ${message} $1
fi
echo $2 | passwd --stdin $1 &>>1.txt
uid=$(awk -F ":" '$1=="'${1}'" {print $3}' /etc/passwd)
gid=$(awk -F ":" '$1=="'${1}'" {print $4}' /etc/passwd)
echo "${1}:${2}" >> passwd.txt
echo "${1}::${uid}:${gid}:${message}:${homeDir}:${shell}" >> user.txt
echo "添加用户成功"
echo
}
add $1 $2 $3
2.用户删除
#!/bin/bash
function del()
{
read -n 1 -p "确认是否要删除该用户y/n:" flag
if [ ${flag} = y ]
then
userdel -r $1 &>>1.txt
sed -i "/$1/d" passwd.txt
sed -i "/$1/d" user.txt
echo
echo -e "删除用户成功\n"
else
echo ""
exit
fi
}
del $1
3.用户修改
#!/bin/bash
function updatePassword()
{
echo
read -p "请输入需要修改的密码:" password
echo ${password} | passwd --stdin $1 &>>1.txt
sed -i "/^${1}/c${1}:${password}" passwd.txt
echo "修改用户密码成功"
echo
}
function updateOther()
{
uid=$(awk -F ":" '$1=="'${1}'" {print $3}' /etc/passwd)
gid=$(awk -F ":" '$1=="'${1}'" {print $4}' /etc/passwd)
homeDir="/home/"$1
shell="/bin/bash"
group=$(awk -F ":" '$3=="'${gid}'" {print $1}' /etc/group)
message=$(awk -F ":" '$1=="'${1}'" {print $5}' /etc/passwd)
password=$(awk -F ":" '$1=="'${1}'" {print $2}' passwd.txt)
echo
read -n 1 -p "是否修改所属组名y/n:" i1
if [ ${i1} = y ]
then
echo
read -p "请输入所属组名:" group
string1=$(awk -F ":" '$1=="'${group}'" {print $1}' /etc/group)
if [ -z "${string1}" ]
then
groupadd ${group}
gid=$(awk -F ":" '$1=="'${group}'" {print $3}' /etc/group)
fi
fi
echo
read -n 1 -p "是否修改目录y/n:" i2
if [ ${i2} = y ]
then
echo
read -p "请输入目录:" homeDir
fi
echo
read -n 1 -p "是否修改用户使用的shelly/n:" i3
if [ ${i3} = y ]
then
echo
read -p "请输入用户使用的shell" shell
fi
echo
read -n 1 -p "是否修改用户描述信息y/n:" i4
if [ ${i4} = y ]
then
echo
read -p "请输入用户描述信息:" message
fi
echo
if [ "${i1}${i2}${i3}${i4}" = "nnnn" ]
then
exit
fi
userdel -r $1
string2=$(awk -F ":" '$1=="'${group}'" {print $1}' /etc/group)
if [ -z "${string2}" ]
then
groupadd ${group}
gid=$(awk -F ":" '$1=="'${group}'" {print $3}' /etc/group)
fi
useradd -d ${homeDir} -s ${shell} -g ${group} -c ${message} $1 &>>1.txt
if [ $? -eq 2 ]
then
useradd -d ${homeDir} -s ${shell} -g ${group} $1
fi
echo ${password} | passwd --stdin $1 &>>1.txt
echo "修改成功"
echo
gid=$(awk -F ":" '$1=="'${group}'" {print $3}' /etc/group)
sed -i "/^${1}/c${1}::${uid}:${gid}:${message}:${homeDir}:${shell}" user.txt
sed -i "/^${1}/c${1}:${password}" passwd.txt
}
if [ $2 -eq 1 ]
then
updatePassword $1
else
updateOther $1
fi
4.用户查询
#!/bin/bash
awk 'NR>19{print $0}' /etc/passwd > user.txt
awk -F ":" 'BEGIN{print"用户名\t UID\t GID\t描述信息\t家目录\t\t shell"}{printf "%-12s%-10s%-10s%-15s%-20s%-20s\n", $1,$3,$4,$5,$6,$7}' user.txt
二、结果显示
 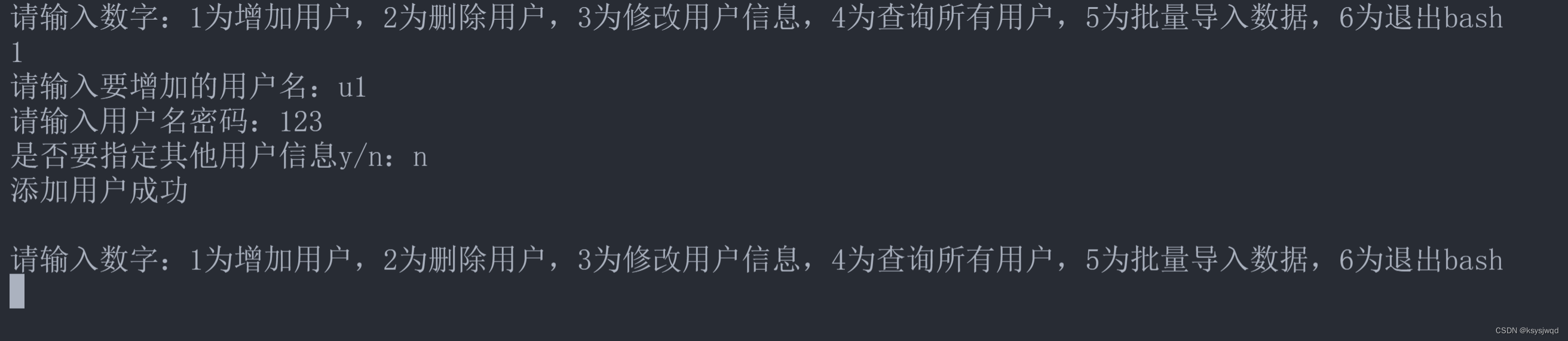 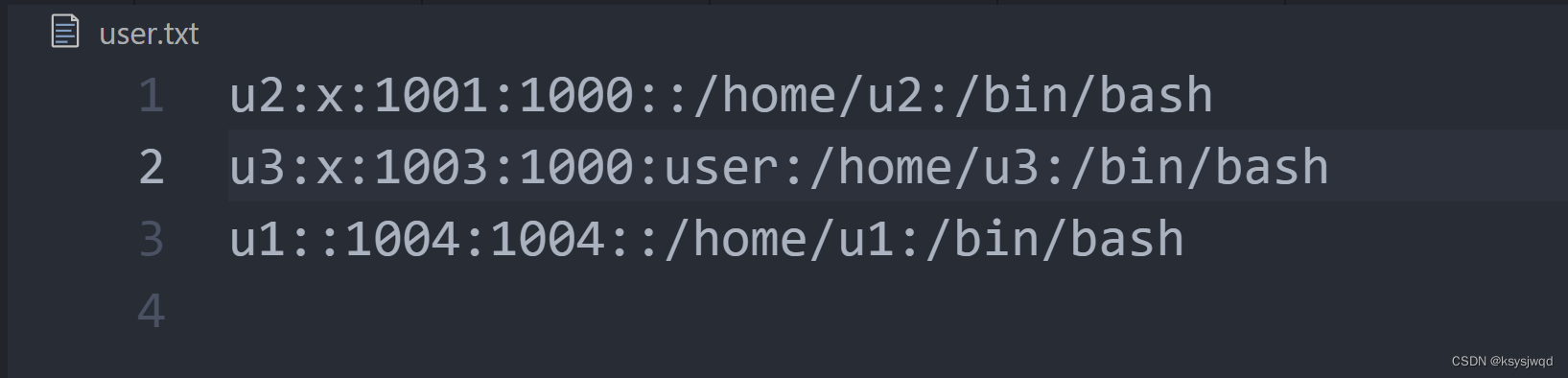  
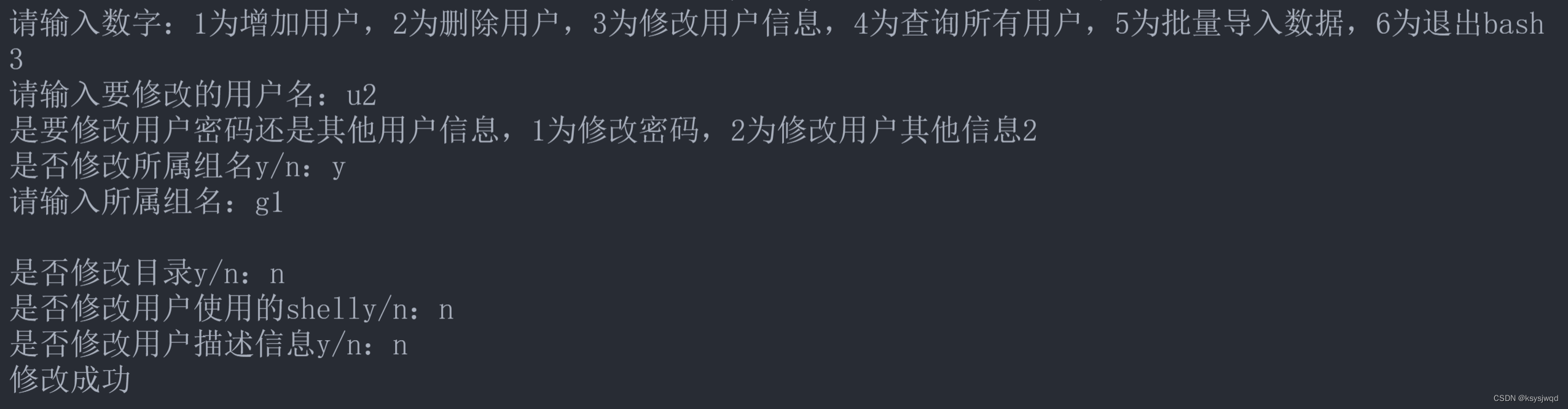 
在相应文件中添加信息来使用批量增加 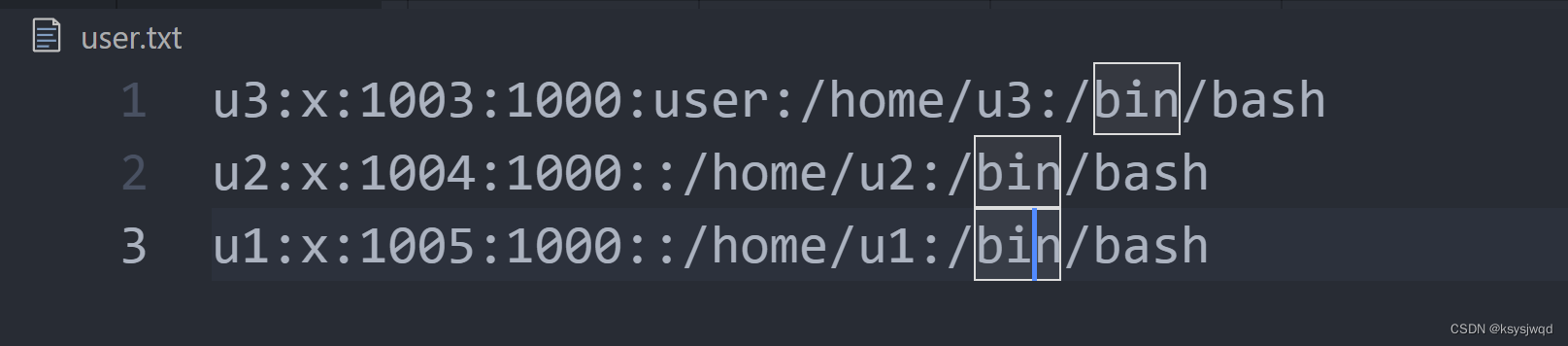 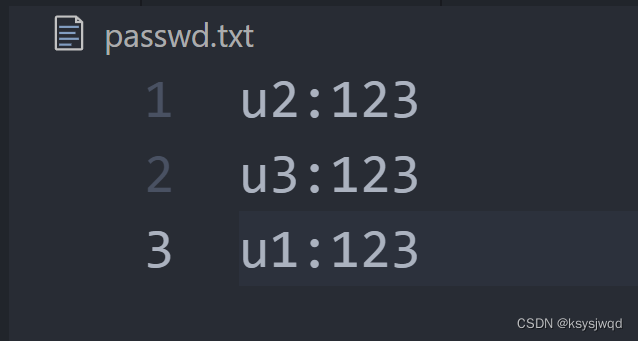 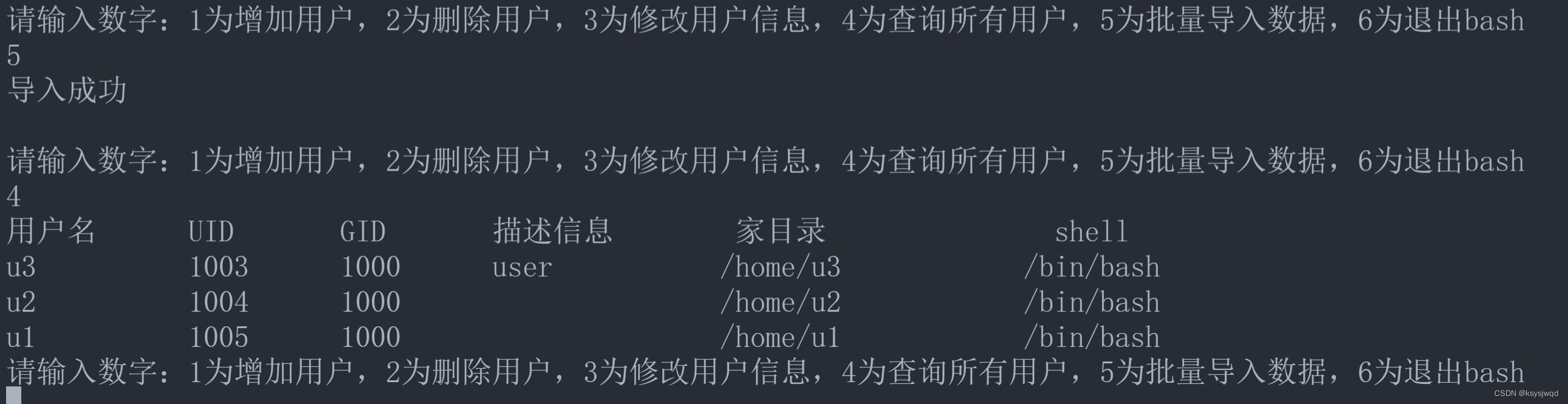
一、使用步骤
使用bash main.sh 运行,在根据提示输入数字来执行相应的功能
代码获取
链接:https://pan.baidu.com/s/1pB9bIiqfKqtmA6MFeY6OgQ?pwd=1122 提取码:1122
|